Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Grundlæggende Operatører
This section explains how to use basic operators in Python.
Aritmetiske Operatorer
Ligesom i andre programmeringssprog kan additions-, subtraktions-, multiplikations- og divisionsoperatorerne bruges med tal.
number = 1 + 2 * 3 / 4.0
print(number)
Prøv at forudsige, hvad svaret vil være. Følger Python rækkefølgen af operationer?
En anden tilgængelig operator er modulo (%) operatoren, som returnerer heltalsresten af divisionen. dividende % divisor = rest.
remainder = 11 % 3
print(remainder)
Ved at bruge to multiplikationssymboler dannes et potensforhold.
squared = 7 ** 2
cubed = 2 ** 3
print(squared)
print(cubed)
Brug af Operatorer med Strenge
Python understøtter sammenkædning af strenge ved brug af additionsoperatoren:
helloworld = "hello" + " " + "world"
print(helloworld)
Python understøtter også multiplikation af strenge for at danne en streng med en gentagende sekvens:
lotsofhellos = "hello" * 10
print(lotsofhellos)
Brug af Operatorer med Lister
Lister kan sammenføjes med additionsoperatoren:
even_numbers = [2,4,6,8]
odd_numbers = [1,3,5,7]
all_numbers = odd_numbers + even_numbers
print(all_numbers)
Ligesom med strenge understøtter Python at danne nye lister med en gentagende sekvens ved brug af multiplikationsoperatoren:
print([1,2,3] * 3)
Øvelse
Målet med denne øvelse er at oprette to lister kaldet x_list
og y_list
, som indeholder 10 forekomster af variablerne x
og y
, henholdsvis. Du skal også oprette en liste kaldet big_list
, som indeholder variablerne x
og y
, 10 gange hver, ved at sammenkæde de to lister, du har oprettet.
x = object()
y = object()
# TODO: change this code
x_list = [x]
y_list = [y]
big_list = []
print("x_list contains %d objects" % len(x_list))
print("y_list contains %d objects" % len(y_list))
print("big_list contains %d objects" % len(big_list))
# testing code
if x_list.count(x) == 10 and y_list.count(y) == 10:
print("Almost there...")
if big_list.count(x) == 10 and big_list.count(y) == 10:
print("Great!")
x = object()
y = object()
# TODO: change this code
x_list = [x] * 10
y_list = [y] * 10
big_list = x_list + y_list
print("x_list contains %d objects" % len(x_list))
print("y_list contains %d objects" % len(y_list))
print("big_list contains %d objects" % len(big_list))
# testing code
if x_list.count(x) == 10 and y_list.count(y) == 10:
print("Almost there...")
if big_list.count(x) == 10 and big_list.count(y) == 10:
print("Great!")
Ex().check_object('x_list').has_equal_value(expr_code = 'len(x_list)')
Ex().check_object('y_list').has_equal_value(expr_code = 'len(y_list)')
Ex().check_object('big_list').has_equal_value(expr_code = 'len(big_list)')
success_msg('Good work!')
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
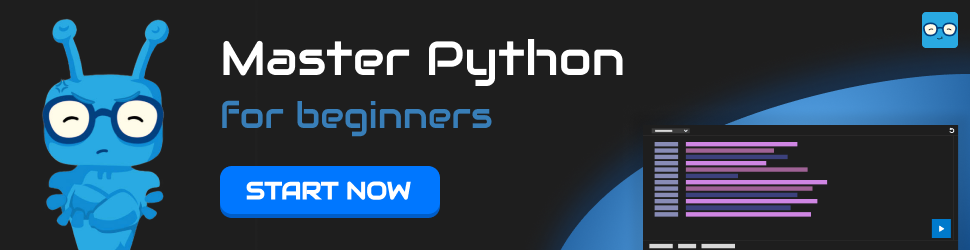