Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Variablen und Typen
Python ist vollständig objektorientiert und nicht "statisch typisiert". Sie müssen Variablen nicht deklarieren, bevor Sie sie verwenden, oder ihren Typ deklarieren. Jede Variable in Python ist ein Objekt.
Dieses Tutorial wird einige grundlegende Typen von Variablen behandeln.
Zahlen
Python unterstützt zwei Arten von Zahlen - Ganzzahlen (ganze Zahlen) und Gleitkommazahlen (Dezimalzahlen). (Es unterstützt auch komplexe Zahlen, die in diesem Tutorial nicht erklärt werden).
Um eine Ganzzahl zu definieren, verwenden Sie die folgende Syntax:
Um eine Gleitkommazahl zu definieren, können Sie eine der folgenden Notationen verwenden:
Zeichenketten
Zeichenketten werden entweder mit einem einfachen oder einem doppelten Anführungszeichen definiert.
Der Unterschied zwischen den beiden ist, dass die Verwendung von doppelten Anführungszeichen es einfach macht, Apostrophe einzuschließen (während diese die Zeichenkette bei der Verwendung von einfachen Anführungszeichen beenden würden).
Es gibt zusätzliche Variationen bei der Definition von Zeichenketten, die es einfacher machen, Dinge wie Wagenrückläufe, Rückwärtsstriche und Unicode-Zeichen einzubeziehen. Diese werden in diesem Tutorial nicht behandelt, sind aber in der Python-Dokumentation behandelt.
Einfache Operatoren können auf Zahlen und Zeichenketten ausgeführt werden:
Zuweisungen können auf mehr als einer Variablen "gleichzeitig" auf derselben Zeile wie folgt vorgenommen werden:
Das Mischen von Operatoren zwischen Zahlen und Zeichenketten wird nicht unterstützt:
Übung
Das Ziel dieser Übung ist es, eine Zeichenkette, eine Ganzzahl und eine Gleitkommazahl zu erstellen. Die Zeichenkette sollte mystring
heißen und das Wort "hello" enthalten. Die Gleitkommazahl sollte myfloat
heißen und die Zahl 10.0 enthalten, und die Ganzzahl sollte myint
heißen und die Zahl 20 enthalten.
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
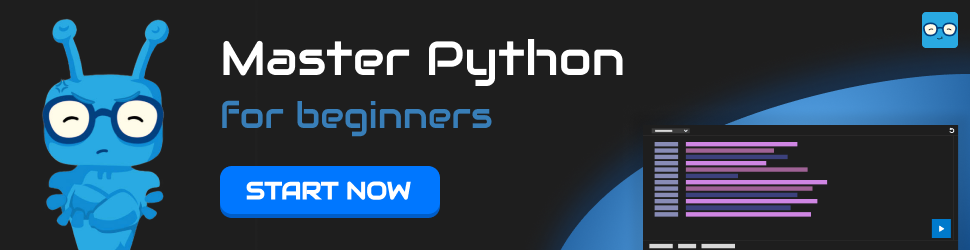