Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Penanganan Pengecualian
When programming, errors happen. It's just a fact of life. Mungkin pengguna memberikan input yang buruk. Mungkin sumber daya jaringan tidak tersedia. Mungkin program kehabisan memori. Atau mungkin programmer bahkan melakukan kesalahan!
Solusi Python terhadap kesalahan adalah pengecualian. Anda mungkin sudah pernah melihat pengecualian sebelumnya.
Ups! Lupa untuk menetapkan nilai ke variabel 'a'.
Tetapi terkadang Anda tidak ingin pengecualian sepenuhnya menghentikan program. Anda mungkin ingin melakukan sesuatu yang spesial ketika pengecualian terjadi. Ini dilakukan dalam blok try/except.
Ini adalah contoh sederhana: Misalkan Anda sedang mengiterasi atas sebuah daftar. Anda perlu mengiterasi atas 20 angka, tetapi daftar ini dibuat dari input pengguna dan mungkin tidak memiliki 20 angka di dalamnya. Setelah Anda mencapai akhir daftar, Anda hanya ingin sisa angka diinterpretasikan sebagai 0. Berikut adalah cara untuk melakukannya:
Nah, itu tidak terlalu sulit! Anda bisa melakukan itu dengan pengecualian apapun. Untuk detail lebih lanjut tentang menangani pengecualian, lihat saja Python Docs
Exercise
Tangani semua pengecualian! Ingat kembali pelajaran sebelumnya untuk mengembalikan nama belakang aktor.
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
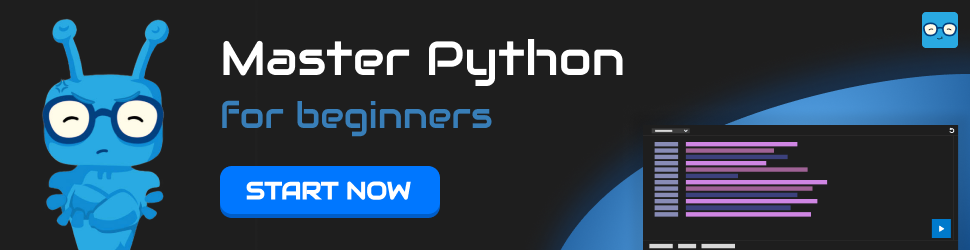