Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Ekspresi Reguler
Regular Expressions (terkadang disingkat menjadi regexp, regex, atau re) adalah alat untuk mencocokkan pola dalam teks. Dalam Python, kita memiliki modul re. Aplikasi untuk ekspresi reguler tersebar luas, tetapi mereka cukup kompleks, jadi ketika mempertimbangkan untuk menggunakan regex untuk tugas tertentu, pikirkan tentang alternatif lain dan datang ke regex sebagai upaya terakhir.
Contoh regex adalah r"^(From|To|Cc).*[email protected]"
. Sekarang untuk penjelasannya:
tanda caret ^
mencocokkan teks di awal baris. Kelompok berikutnya, bagian dengan (From|To|Cc)
berarti bahwa baris harus dimulai dengan salah satu kata yang dipisahkan oleh pipa |
. Itu disebut operator OR, dan regex akan cocok jika baris dimulai dengan salah satu kata dalam kelompok tersebut. .*?
berarti mencocokkan secara tidak serakah dengan jumlah karakter apa pun, kecuali karakter newline \n
. Bagian tidak serakah berarti mencocokkan sedikit pengulangan mungkin. Karakter .
berarti karakter apa pun yang bukan newline, *
berarti mengulangi 0 atau lebih kali, dan karakter ?
membuatnya tidak serakah.
Jadi, baris berikut akan dicocokkan oleh regex tersebut:
From: [email protected]
To: !asp]<,. [email protected]
Referensi lengkap untuk sintaks re tersedia di dokumentasi python.
Sebagai contoh regex untuk mencocokkan email yang "tepat" (seperti yang ada dalam latihan), lihat ini
# Example:
import re
pattern = re.compile(r"\[(on|off)\]") # Slight optimization
print(re.search(pattern, "Mono: Playback 65 [75%] [-16.50dB] [on]"))
# Returns a Match object!
print(re.search(pattern, "Nada...:-("))
# Doesn't return anything.
# End Example
# Exercise: make a regular expression that will match an email
def test_email(your_pattern):
pattern = re.compile(your_pattern)
emails = ["[email protected]", "[email protected]", "wha.t.`1an?ug{}[email protected]"]
for email in emails:
if not re.match(pattern, email):
print("You failed to match %s" % (email))
elif not your_pattern:
print("Forgot to enter a pattern!")
else:
print("Pass")
pattern = r"" # Your pattern here!
test_email(pattern)
# Exercise: make a regular expression that will match an email
import re
def test_email(your_pattern):
pattern = re.compile(your_pattern)
emails = ["[email protected]", "[email protected]", "wha.t.`1an?ug{}[email protected]"]
for email in emails:
if not re.match(pattern, email):
print("You failed to match %s" % (email))
elif not your_pattern:
print("Forgot to enter a pattern!")
else:
print("Pass")
# Your pattern here!
pattern = r"\"?([-a-zA-Z0-9.`?{}]+@\w+\.\w+)\"?"
test_email(pattern)
test_output_contains("Pass")
success_msg("Great work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
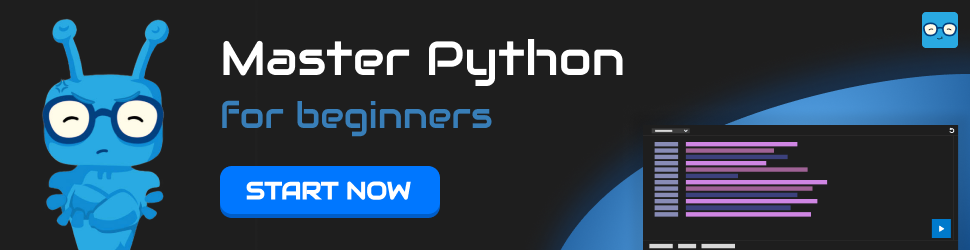