Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Variabler og typer
Python er fullstendig objektorientert, og ikke "statisk typet". Du trenger ikke å erklære variabler før du bruker dem, eller erklære deres type. Hver variabel i Python er et objekt.
Denne veiledningen vil dekke noen grunnleggende typer variabler.
Tall
Python støtter to typer tall - heltall (hele tall) og flyttall (desimaltall). (Det støtter også komplekse tall, som ikke vil bli forklart i denne veiledningen).
For å definere et heltall, bruk følgende syntaks:
myint = 7
print(myint)
For å definere en flyttall, kan du bruke en av følgende notasjoner:
myfloat = 7.0
print(myfloat)
myfloat = float(7)
print(myfloat)
Strenger
Strenger kan defineres enten med et enkelt anførselstegn eller et dobbelt anførselstegn.
mystring = 'hello'
print(mystring)
mystring = "hello"
print(mystring)
Forskjellen mellom de to er at ved å bruke dobbelt anførselstegn er det enkelt å inkludere apostrofer (mens disse ville avslutte strengen hvis du brukte enkelt anførselstegn)
mystring = "Don't worry about apostrophes"
print(mystring)
Det er flere varianter av hvordan man definerer strenger som gjør det enklere å inkludere ting som linjeskift, bakstreker og Unicode-tegn. Disse ligger utenfor omfanget av denne veiledningen, men er dekket i Python-dokumentasjonen.
Enkle operasjoner kan utføres på tall og strenger:
one = 1
two = 2
three = one + two
print(three)
hello = "hello"
world = "world"
helloworld = hello + " " + world
print(helloworld)
Tilordninger kan gjøres på mer enn en variabel "samtidig" på samme linje slik som dette:
a, b = 3, 4
print(a, b)
Å blande operatører mellom tall og strenger støttes ikke:
# Dette vil ikke fungere!
one = 1
two = 2
hello = "hello"
print(one + two + hello)
Øvelse
Målet med denne øvelsen er å lage en streng, et heltall, og et flyttall. Strengen skal hete mystring
og inneholde ordet "hello". Flyttallet skal hete myfloat
og inneholde tallet 10.0, og heltallet skal hete myint
og inneholde tallet 20.
# change this code
mystring = None
myfloat = None
myint = None
# testing code
if mystring == "hello":
print("String: %s" % mystring)
if isinstance(myfloat, float) and myfloat == 10.0:
print("Float: %f" % myfloat)
if isinstance(myint, int) and myint == 20:
print("Integer: %d" % myint)
# change this code
mystring = "hello"
myfloat = 10.0
myint = 20
# testing code
if mystring == "hello":
print("String: %s" % mystring)
if isinstance(myfloat, float) and myfloat == 10.0:
print("Float: %f" % myfloat)
if isinstance(myint, int) and myint == 20:
print("Integer: %d" % myint)
test_object('mystring', incorrect_msg="Don't forget to change `mystring` to the correct value from the exercise description.")
test_object('myfloat', incorrect_msg="Don't forget to change `myfloat` to the correct value from the exercise description.")
test_object('myint', incorrect_msg="Don't forget to change `myint` to the correct value from the exercise description.")
test_output_contains("String: hello",no_output_msg= "Make sure your string matches exactly to the exercise desciption.")
test_output_contains("Float: 10.000000",no_output_msg= "Make sure your float matches exactly to the exercise desciption.")
test_output_contains("Integer: 20",no_output_msg= "Make sure your integer matches exactly to the exercise desciption.")
success_msg("Great job!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
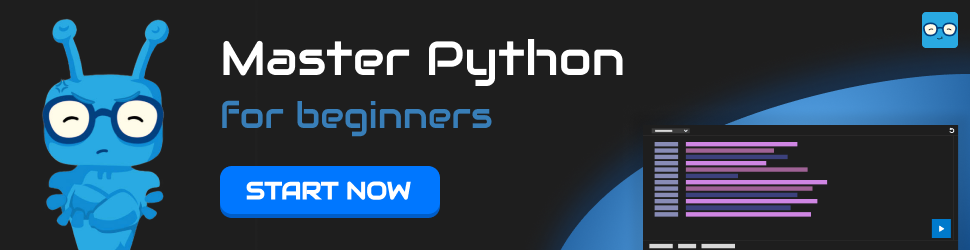