Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Klasy i Obiekty
Obiekty są enkapsulacją zmiennych i funkcji w jedną jednostkę. Obiekty otrzymują swoje zmienne i funkcje z klas. Klasy to zasadniczo szablon do tworzenia obiektów.
Bardzo podstawowa klasa wyglądałaby tak:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
Wyjaśnimy, dlaczego musisz dołączyć "self" jako parametr nieco później. Najpierw, aby przypisać powyższą klasę (szablon) do obiektu, należy zrobi jeden z poniższych kroków:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
Teraz zmienna "myobjectx" przechowuje obiekt klasy "MyClass", która zawiera zmienną i funkcję zdefiniowaną wewnątrz klasy o nazwie "MyClass".
Dostęp do zmiennych obiektu
Aby uzyskać dostęp do zmiennej wewnątrz nowo utworzonego obiektu "myobjectx", należy wykonać następujące kroki:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
myobjectx.variable
Na przykład poniżej kod wypisze ciąg "blah":
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
print(myobjectx.variable)
Możesz utworzyć wiele różnych obiektów tej samej klasy (mają tych samych zmiennych i funkcji zdefiniowanych). Jednakże, każdy obiekt zawiera niezależne kopie zmiennych zdefiniowanych w klasie. Na przykład, jeśli zdefiniujemy inny obiekt za pomocą klasy "MyClass", a następnie zmienimy ciąg w zmiennej:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
myobjecty = MyClass()
myobjecty.variable = "yackity"
# Następnie wypiszemy obie wartości
print(myobjectx.variable)
print(myobjecty.variable)
Dostęp do funkcji obiektu
Aby uzyskać dostęp do funkcji wewnątrz obiektu, używasz notacji podobnej do uzyskiwania dostępu do zmiennej:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
myobjectx.function()
Powyższy kod wypisze komunikat: "This is a message inside the class."
init()
Funkcja __init__()
, to specjalna funkcja, która jest wywoływana, gdy klasa jest inicjowana. Jest używana do przypisywania wartości w klasie.
class NumberHolder:
def __init__(self, number):
self.number = number
def returnNumber(self):
return self.number
var = NumberHolder(7)
print(var.returnNumber()) # Wypisze '7'
Ćwiczenie
Mamy zdefiniowaną klasę dla pojazdów. Utwórz dwa nowe pojazdy o nazwach car1 i car2. Ustaw car1 na czerwoną kabriolet wartej 60,000.00 $ z nazwą Fer, a car2 na niebieski van o nazwie Jump wart 10,000.00 $.
# define the Vehicle class
class Vehicle:
name = ""
kind = "car"
color = ""
value = 100.00
def description(self):
desc_str = "%s is a %s %s worth $%.2f." % (self.name, self.color, self.kind, self.value)
return desc_str
# your code goes here
# test code
print(car1.description())
print(car2.description())
# define the Vehicle class
class Vehicle:
name = ""
kind = "car"
color = ""
value = 100.00
def description(self):
desc_str = "%s is a %s %s worth $%.2f." % (self.name, self.color, self.kind, self.value)
return desc_str
# your code goes here
car1 = Vehicle()
car1.name = "Fer"
car1.color = "red"
car1.kind = "convertible"
car1.value = 60000.00
car2 = Vehicle()
car2.name = "Jump"
car2.color = "blue"
car2.kind = "van"
car2.value = 10000.00
# test code
print(car1.description())
print(car2.description())
#test_output_contains('Fer is a red convertible worth $60000.00.')
#test_output_contains('Jump is a blue van worth $10000.00.')
success_msg("Great job!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
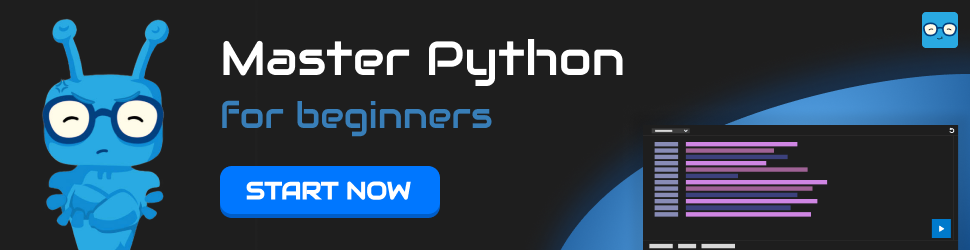