Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Condiții
Python folosește logica booleană pentru a evalua condițiile. Valorile booleene True și False sunt returnate atunci când o expresie este comparată sau evaluată. De exemplu:
x = 2 print(x == 2) # prints out True print(x == 3) # prints out False print(x < 3) # prints out True
Observați că atribuirea de variabile se face folosind un singur operator egal "=", în timp ce comparația între două variabile se face folosind operatorul dublu egal "==". Operatorul "diferență" este marcat ca "!=".
Operatorii booleani
Operatorii booleani "and" și "or" permit construirea de expresii booleene complexe, de exemplu:
name = "John" age = 23 if name == "John" and age == 23: print("Your name is John, and you are also 23 years old.")
if name == "John" or name == "Rick": print("Your name is either John or Rick.")
Operatorul "in"
Operatorul "in" poate fi folosit pentru a verifica dacă un obiect specificat există într-un container de obiecte iterabile, cum ar fi o listă:
name = "John" if name in ["John", "Rick"]: print("Your name is either John or Rick.")
Python folosește indentarea pentru a defini blocurile de cod, în loc de acolade. Indentarea standard în Python este de 4 spații, deși taburile și orice altă dimensiune a spațiului vor funcționa, atâta timp cât este consecventă. Observați că blocurile de cod nu au nevoie de nicio terminare.
Iată un exemplu de utilizare a instrucțiunii "if" în Python folosind blocuri de cod:
statement = False another_statement = True if statement is True: # do something pass elif another_statement is True: # else if # do something else pass else: # do another thing pass
De exemplu:
x = 2 if x == 2: print("x equals two!") else: print("x does not equal to two.")
O instrucțiune este evaluată ca adevărată dacă unul dintre următoarele este corect: 1. Variabila booleană "True" este dată sau calculată folosind o expresie, cum ar fi o comparație aritmetică. 2. Un obiect care nu este considerat "gol" este transmis.
Iată câteva exemple de obiecte care sunt considerate ca fiind goale: 1. Un șir de caractere gol: "" 2. O listă goală: [] 3. Numărul zero: 0 4. Variabila booleană falsă: False
Operatorul 'is'
Spre deosebire de operatorul dublu egal "==", operatorul "is" nu compară valorile variabilelor, ci însăși instanțele. De exemplu:
x = [1,2,3] y = [1,2,3] print(x == y) # Prints out True print(x is y) # Prints out False
Operatorul "not"
Utilizarea "not" înainte de o expresie booleană o inversează:
print(not False) # Prints out True print((not False) == (False)) # Prints out False
Exercițiu
Schimbați variabilele din prima secțiune, astfel încât fiecare instrucțiune "if" să se rezolve ca True.
# change this code
number = 10
second_number = 10
first_array = []
second_array = [1,2,3]
if number > 15:
print("1")
if first_array:
print("2")
if len(second_array) == 2:
print("3")
if len(first_array) + len(second_array) == 5:
print("4")
if first_array and first_array[0] == 1:
print("5")
if not second_number:
print("6")
# change this code
number = 16
second_number = 0
first_array = [1,2,3]
second_array = [1,2]
if number > 15:
print("1")
if first_array:
print("2")
if len(second_array) == 2:
print("3")
if len(first_array) + len(second_array) == 5:
print("4")
if first_array and first_array[0] == 1:
print("5")
if not second_number:
print("6")
test_output_contains("1", no_output_msg= "Did you print out 1 if `number` is greater than 15?")
test_output_contains("2", no_output_msg= "Did you print out 2 if there exists a list `first_array`?")
test_output_contains("3", no_output_msg= "Did you print out 3 if the length of `second_array` is 2?")
test_output_contains("4", no_output_msg= "Did you print out 4 if len(first_array) + len(second_array) == 5?")
test_output_contains("5", no_output_msg= "Did you print out 5 if first_array and first_array[0] == 1?")
test_output_contains("6", no_output_msg= "Did you print out 6 if not second_number?")
success_msg("Great Work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
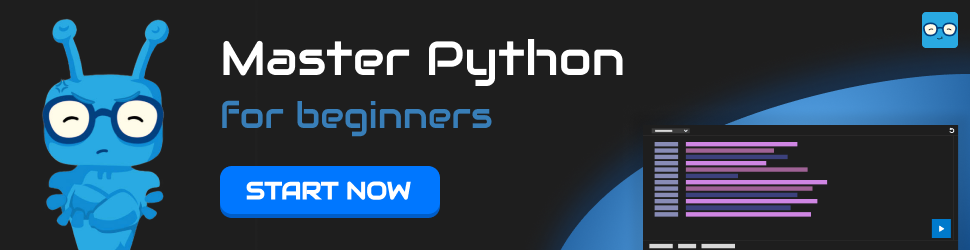