Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Променливи и типове
Python е напълно обектно ориентиран и не е „статично типизиран“. Не е необходимо да декларирате променливи преди да ги използвате или да декларирате техния тип. Всяка променлива в Python е обект.
Този урок ще разгледа няколко основни типа променливи.
Numbers
Python поддържа два типа числа - цели (целочислени) и числа с плаваща запетая (десетични). (Също така поддържа комплексни числа, които няма да бъдат обяснени в този урок).
За да дефинирате цяло число, използвайте следния синтаксис:
myint = 7
print(myint)
За да дефинирате число с плаваща запетая, можете да използвате някоя от следните нотации:
myfloat = 7.0
print(myfloat)
myfloat = float(7)
print(myfloat)
Strings
Низовете се дефинират или с единични кавички, или с двойни кавички.
mystring = 'hello'
print(mystring)
mystring = "hello"
print(mystring)
Разликата между двете е, че използването на двойни кавички улеснява включването на апострофи (докато те биха прекъснали низа, ако използвате единични кавички)
mystring = "Don't worry about apostrophes"
print(mystring)
Има допълнителни вариации при дефинирането на низове, които улесняват включването на неща като преводи на каретката, наклонени черти и Unicode символи. Те са извън обхвата на този урок, но са разгледани в Python документацията.
Прости оператори могат да бъдат изпълнявани върху числа и низове:
one = 1
two = 2
three = one + two
print(three)
hello = "hello"
world = "world"
helloworld = hello + " " + world
print(helloworld)
Присвояването може да се направи на повече от една променлива „едновременно“ на същия ред така:
a, b = 3, 4
print(a, b)
Смесването на оператори между числа и низове не се поддържа:
# Това няма да работи!
one = 1
two = 2
hello = "hello"
print(one + two + hello)
Упражнение
Целта на това упражнение е да създадете низ, цяло число и число с плаваща запетая. Низът трябва да се казва mystring
и да съдържа думата "hello". Числото с плаваща запетая трябва да се казва myfloat
и да съдържа числото 10.0, а цялото число трябва да се казва myint
и да съдържа числото 20.
# change this code
mystring = None
myfloat = None
myint = None
# testing code
if mystring == "hello":
print("String: %s" % mystring)
if isinstance(myfloat, float) and myfloat == 10.0:
print("Float: %f" % myfloat)
if isinstance(myint, int) and myint == 20:
print("Integer: %d" % myint)
# change this code
mystring = "hello"
myfloat = 10.0
myint = 20
# testing code
if mystring == "hello":
print("String: %s" % mystring)
if isinstance(myfloat, float) and myfloat == 10.0:
print("Float: %f" % myfloat)
if isinstance(myint, int) and myint == 20:
print("Integer: %d" % myint)
test_object('mystring', incorrect_msg="Don't forget to change `mystring` to the correct value from the exercise description.")
test_object('myfloat', incorrect_msg="Don't forget to change `myfloat` to the correct value from the exercise description.")
test_object('myint', incorrect_msg="Don't forget to change `myint` to the correct value from the exercise description.")
test_output_contains("String: hello",no_output_msg= "Make sure your string matches exactly to the exercise desciption.")
test_output_contains("Float: 10.000000",no_output_msg= "Make sure your float matches exactly to the exercise desciption.")
test_output_contains("Integer: 20",no_output_msg= "Make sure your integer matches exactly to the exercise desciption.")
success_msg("Great job!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
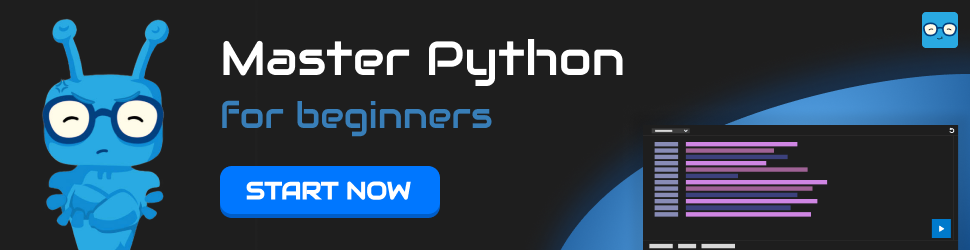