Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Základní operátory
This section explains how to use basic operators in Python.
Aritmetické operátory
Stejně jako v jiných programovacích jazycích lze operátory pro sčítání, odčítání, násobení a dělení používat s čísly.
Zkuste odhadnout, jaká bude odpověď. Dodržuje python pořadí operací?
Další dostupný operátor je modulo (%) operátor, který vrací celočíselný zbytek dělení. dividend % divisor = zbytek.
Použití dvou symbolů násobení vytváří mocninný vztah.
Použití operátorů s řetězci
Python podporuje spojování řetězců pomocí sčítacího operátoru:
Python také podporuje násobení řetězců k vytvoření řetězce s opakující se sekvencí:
Použití operátorů se seznamy
Seznamy mohou být spojeny pomocí sčítacího operátoru:
Stejně jako u řetězců, Python podporuje vytvoření nových seznamů s opakující se sekvencí pomocí násobícího operátoru:
Cvičení
Cílem tohoto cvičení je vytvořit dva seznamy nazvané x_list
a y_list
, které obsahují 10 instance proměnných x
a y
, respektive. Je také požadováno vytvořit seznam nazvaný big_list
, který obsahuje proměnné x
a y
10krát každou, spojením dvou seznamů, které jste vytvořili.
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
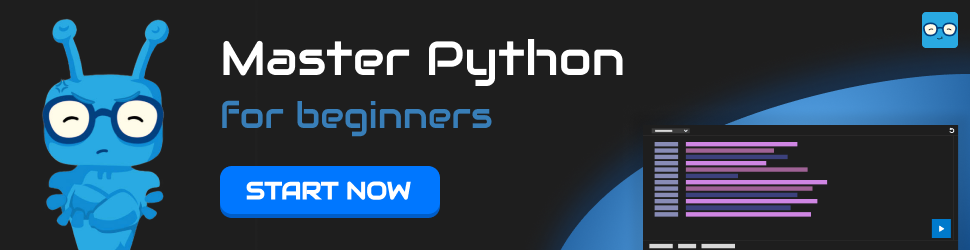