Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Funkce
Co jsou funkce?
Funkce jsou pohodlný způsob, jak rozdělit váš kód na užitečné bloky, což nám umožňuje uspořádat náš kód, učinit ho čitelnějším, znovu použít a ušetřit čas. Také funkce jsou klíčovým způsobem, jak definovat rozhraní, takže programátoři mohou sdílet svůj kód.
Jak píšete funkce v Pythonu?
Jak jsme viděli v předchozích tutoriálech, Python využívá bloky.
Blok je oblast kódu napsaná ve formátu:
Kde řádek bloku je více Python kódu (dokonce další blok) a hlava bloku je ve formátu: block_keyword block_name(argument1,argument2, ...) Klíčová slova, která již znáte, jsou "if", "for", a "while".
Funkce v Pythonu jsou definovány pomocí klíčového slova "def", následovaného názvem funkce jako názvem bloku. Například:
Funkce mohou také přijímat argumenty (proměnné předané volajícímu funkce). Například:
Funkce mohou vrátit hodnotu volajícímu pomocí klíčového slova 'return'. Například:
Jak voláte funkce v Pythonu?
Jednoduše napište jméno funkce následované (), umístěním jakýchkoliv potřebných argumentů do závorek. Například, zavolejme funkce napsané výše (v předchozím příkladu):
Cvičení
V tomto cvičení použijete existující funkci a přidáte vlastní funkci pro vytvoření plně funkčního programu.
-
Přidejte funkci s názvem
list_benefits()
, která vrátí následující seznam řetězců: "Více organizovaný kód", "Čitelnější kód", "Snazší opětovné použití kódu", "Umožňuje programátorům sdílet a propojit kód dohromady" -
Přidejte funkci s názvem
build_sentence(info)
, která přijímá jediný argument obsahující řetězec a vrací větu začínající daným řetězcem a končící řetězcem " je výhoda funkcí!" -
Spusťte a uvidíte, jak všechny funkce pracují společně!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
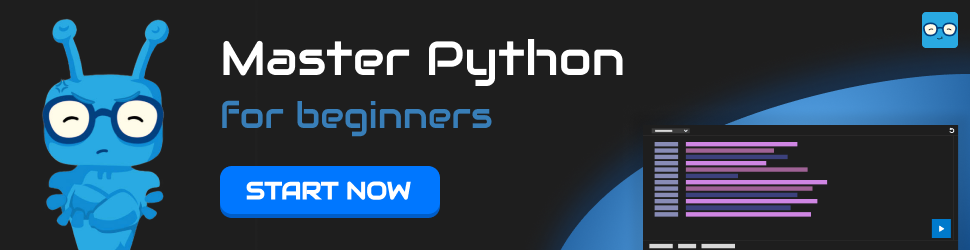