Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
ตัวดำเนินการพื้นฐาน
This section explains how to use basic operators in Python.
ตัวดำเนินการทางคณิตศาสตร์
เช่นเดียวกับภาษาโปรแกรมอื่นๆ ตัวดำเนินการบวก ลบ คูณ และหารสามารถใช้กับตัวเลขได้
number = 1 + 2 * 3 / 4.0
print(number)
ลองทำนายคำตอบว่าคืออะไร Python ใช้ลำดับความสำคัญในการดำเนินการหรือไม่?
อีกตัวดำเนินการหนึ่งที่มีให้ใช้คือตัวดำเนินการโมดูโล (%) ซึ่งจะส่งคืนเศษของการหาร dividend % divisor = remainder.
remainder = 11 % 3
print(remainder)
การใช้สัญลักษณ์การคูณสองครั้งทำให้เกิดความสัมพันธ์ของการยกกำลัง
squared = 7 ** 2
cubed = 2 ** 3
print(squared)
print(cubed)
การใช้ตัวดำเนินการกับสตริง
Python สนับสนุนการต่อสตริงโดยใช้ตัวดำเนินการบวก:
helloworld = "hello" + " " + "world"
print(helloworld)
Python ยังสนับสนุนการคูณสตริงเพื่อสร้างสตริงที่มีลำดับการทำซ้ำ:
lotsofhellos = "hello" * 10
print(lotsofhellos)
การใช้ตัวดำเนินการกับรายการ
รายการสามารถจับคู่กันได้ด้วยตัวดำเนินการบวก:
even_numbers = [2,4,6,8]
odd_numbers = [1,3,5,7]
all_numbers = odd_numbers + even_numbers
print(all_numbers)
เช่นเดียวกับในสตริง Python สนับสนุนการสร้างรายการใหม่ที่มีลำดับการทำซ้ำโดยใช้ตัวดำเนินการคูณ:
print([1,2,3] * 3)
Exercise
The target of this exercise is to create two lists called x_list
and y_list
,
which contain 10 instances of the variables x
and y
, respectively.
You are also required to create a list called big_list
, which contains
the variables x
and y
, 10 times each, by concatenating the two lists you have created.
x = object()
y = object()
# TODO: change this code
x_list = [x]
y_list = [y]
big_list = []
print("x_list contains %d objects" % len(x_list))
print("y_list contains %d objects" % len(y_list))
print("big_list contains %d objects" % len(big_list))
# testing code
if x_list.count(x) == 10 and y_list.count(y) == 10:
print("Almost there...")
if big_list.count(x) == 10 and big_list.count(y) == 10:
print("Great!")
x = object()
y = object()
# TODO: change this code
x_list = [x] * 10
y_list = [y] * 10
big_list = x_list + y_list
print("x_list contains %d objects" % len(x_list))
print("y_list contains %d objects" % len(y_list))
print("big_list contains %d objects" % len(big_list))
# testing code
if x_list.count(x) == 10 and y_list.count(y) == 10:
print("Almost there...")
if big_list.count(x) == 10 and big_list.count(y) == 10:
print("Great!")
Ex().check_object('x_list').has_equal_value(expr_code = 'len(x_list)')
Ex().check_object('y_list').has_equal_value(expr_code = 'len(y_list)')
Ex().check_object('big_list').has_equal_value(expr_code = 'len(big_list)')
success_msg('Good work!')
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
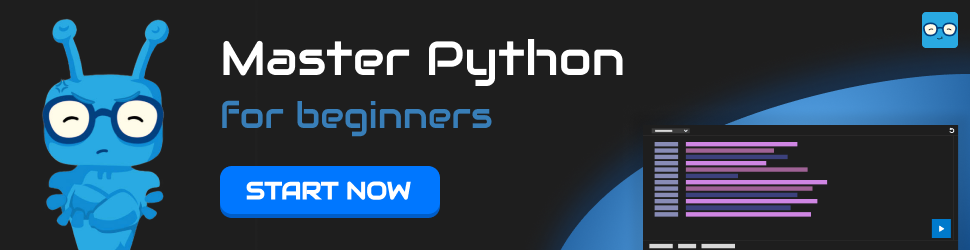