Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
เงื่อนไข
Python ใช้ตรรกะ boolean ในการประเมินเงื่อนไข ค่า boolean True และ False จะถูกส่งคืนเมื่อมีการเปรียบเทียบหรือประเมินนิพจน์ ตัวอย่างเช่น:
x = 2 print(x == 2) # พิมพ์ค่าออกมาเป็น True print(x == 3) # พิมพ์ค่าออกมาเป็น False print(x < 3) # พิมพ์ค่าออกมาเป็น True
สังเกตว่าการกำหนดค่าตัวแปรจะใช้เครื่องหมายเท่ากับเดี่ยว "=" ในขณะที่การเปรียบเทียบระหว่างตัวแปรสองตัวจะใช้เครื่องหมายเท่ากับคู่ "==" ส่วนนิพจน์ "ไม่เท่ากับ" จะใช้เครื่องหมาย "!="
ตัวดำเนินการ Boolean
ตัวดำเนินการ boolean "and" และ "or" อนุญาตให้สร้างนิพจน์ boolean ที่ซับซ้อนได้ เช่น:
name = "John" age = 23 if name == "John" and age == 23: print("Your name is John, and you are also 23 years old.")
if name == "John" or name == "Rick": print("Your name is either John or Rick.")
ตัวดำเนินการ "in"
ตัวดำเนินการ "in" สามารถใช้ตรวจสอบว่ามีวัตถุที่กำหนดอยู่ภายในวัตถุที่เป็นออบเจกต์แบบ iterable เช่น รายการหรือไม่:
name = "John" if name in ["John", "Rick"]: print("Your name is either John or Rick.")
Python ใช้การเว้นวรรคเพื่อกำหนดกลุ่มโค้ด แทนการใช้วงเล็บ การเว้นวรรคมาตรฐานใน Python คือ 4 ช่อง ถึงแม้ว่าจะใช้แท็บหรือขนาดช่องว่างแบบอื่นได้ ตราบใดที่คงความสม่ำเสมอเอาไว้ สังเกตว่ากลุ่มโค้ดไม่จำเป็นต้องมีจุดสิ้นสุด
ต่อไปนี้คือตัวอย่างการใช้คำสั่ง "if" ของ Python โดยใช้กลุ่มโค้ด:
statement = False another_statement = True if statement is True: # ทำบางอย่าง pass elif another_statement is True: # else if # ทำอีกอย่างหนึ่ง pass else: # ทำอีกสิ่งหนึ่ง pass
ตัวอย่างเช่น:
x = 2 if x == 2: print("x equals two!") else: print("x does not equal to two.")
นิพจน์จะถูกประเมินว่าเป็นจริงหากถูกต้องตามหนึ่งในกรณีต่อไปนี้: 1. ให้ตัวแปร boolean "True" หรือนิพจน์ที่คำนวณให้เป็นจริง เช่น การเปรียบเทียบทางคณิตศาสตร์ 2. มีการส่งอ็อบเจกต์ที่ไม่ถือว่า "ว่างเปล่า"
นี่คือตัวอย่างอ็อบเจกต์ที่ถือว่าเป็นว่างเปล่า: 1. สตริงว่าง: "" 2. รายการว่าง: [] 3. ค่าเลขศูนย์: 0 4. ตัวแปร boolean false: False
ตัวดำเนินการ 'is'
ไม่เหมือนกับตัวดำเนินการเท่ากับคู่ "==" ตัวดำเนินการ "is" ไม่ได้ใช้เปรียบเทียบค่าของตัวแปรแต่ใช้เปรียบเทียบออบเจกต์เอง ตัวอย่างเช่น:
x = [1,2,3] y = [1,2,3] print(x == y) # พิมพ์ค่าออกมาเป็น True print(x is y) # พิมพ์ค่าออกมาเป็น False
ตัวดำเนินการ "not"
การใช้ "not" ก่อนนิพจน์ boolean จะทำให้มันกลับค่า:
print(not False) # พิมพ์ค่าออกมาเป็น True print((not False) == (False)) # พิมพ์ค่าออกมาเป็น False
Exercise
เปลี่ยนค่าตัวแปรในส่วนแรกเพื่อให้คำสั่ง if แต่ละคำสั่งเป็นจริง
# change this code
number = 10
second_number = 10
first_array = []
second_array = [1,2,3]
if number > 15:
print("1")
if first_array:
print("2")
if len(second_array) == 2:
print("3")
if len(first_array) + len(second_array) == 5:
print("4")
if first_array and first_array[0] == 1:
print("5")
if not second_number:
print("6")
# change this code
number = 16
second_number = 0
first_array = [1,2,3]
second_array = [1,2]
if number > 15:
print("1")
if first_array:
print("2")
if len(second_array) == 2:
print("3")
if len(first_array) + len(second_array) == 5:
print("4")
if first_array and first_array[0] == 1:
print("5")
if not second_number:
print("6")
test_output_contains("1", no_output_msg= "Did you print out 1 if `number` is greater than 15?")
test_output_contains("2", no_output_msg= "Did you print out 2 if there exists a list `first_array`?")
test_output_contains("3", no_output_msg= "Did you print out 3 if the length of `second_array` is 2?")
test_output_contains("4", no_output_msg= "Did you print out 4 if len(first_array) + len(second_array) == 5?")
test_output_contains("5", no_output_msg= "Did you print out 5 if first_array and first_array[0] == 1?")
test_output_contains("6", no_output_msg= "Did you print out 6 if not second_number?")
success_msg("Great Work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
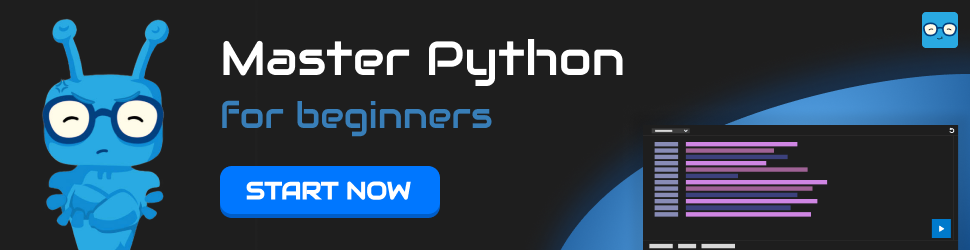