Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
生成器
生成器很容易实现,但理解起来有些困难。
生成器用于创建迭代器,但采用了不同的方法。生成器是一些简单的函数,以一种特殊的方式一次返回一个可迭代的项目集合。
当使用 for 语句开始对一组项目进行迭代时,生成器就会运行。一旦生成器的函数代码达到 "yield" 语句,生成器就会将执行权交还给 for 循环,从集合中返回一个新值。生成器函数可以根据需要生成任意多个值(可能是无限的),并在每次轮到它时产生一个。
这是一个简单的生成器函数示例,它返回 7 个随机整数:
```python import random
def lottery(): # 返回 6 个介于 1 到 40 之间的数字 for i in range(6): yield random.randint(1, 40)
# 返回第 7 个介于 1 到 15 之间的数字
yield random.randint(1, 15)
for random_number in lottery(): print("And the next number is... %d!" % (random_number)) ```
这个函数自行决定如何生成随机数,并逐一执行 yield 语句,在此期间暂停以将执行权交还给主 for 循环。
Exercise
编写一个生成器函数,返回斐波那契数列。它们是使用以下公式计算的:数列的前两个数字总是等于 1,每返回一个连续的数字都是最后两个数字的和。
提示:你可以在生成器函数中只使用两个变量吗?记住,可以同时进行赋值。代码
python
a = 1
b = 2
a, b = b, a
print(a, b)
将同时交换 a 和 b 的值。
# fill in this function
def fib():
pass #this is a null statement which does nothing when executed, useful as a placeholder.
# testing code
import types
if type(fib()) == types.GeneratorType:
print("Good, The fib function is a generator.")
counter = 0
for n in fib():
print(n)
counter += 1
if counter == 10:
break
# fill in this function
def fib():
a, b = 1, 1
while 1:
yield a
a, b = b, a + b
# testing code
import types
if type(fib()) == types.GeneratorType:
print("Good, The fib function is a generator.")
counter = 0
for n in fib():
print(n)
counter += 1
if counter == 10:
break
test_output_contains("Good, The fib function is a generator.")
success_msg('Good work!')
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
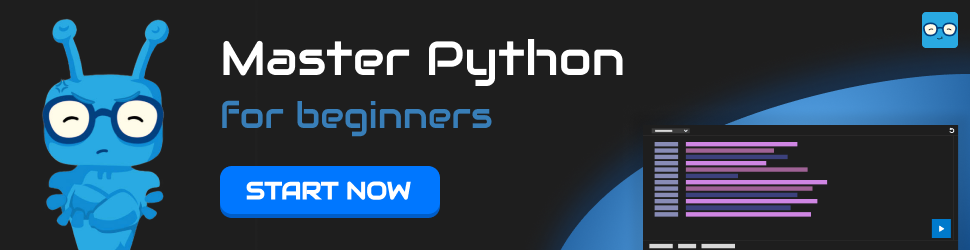