Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
正则表达式
正则表达式(有时缩写为regexp, regex, 或 re)是一种用于匹配文本模式的工具。在Python中,我们有re模块。正则表达式的应用范围很广,但它们相当复杂,因此在考虑使用正则表达式处理某个任务时,先考虑其他替代方案,最后再选择使用正则表达式。
一个正则表达式的示例是 r"^(From|To|Cc).*[email protected]"
现在解释一下:
插入符号 ^
匹配行首的文本。接下来的组,即包含(From|To|Cc)
的部分表示行必须以用管道符号|
分开的词之一开头。这称为OR运算符,并且如果行以组中的任何单词开头,则正则表达式将匹配。.*?
表示非贪婪地匹配除换行符\n
之外的任意数量的字符。非贪婪部分意味着尽可能少地匹配重复。.
字符表示任何非换行字符,*
表示重复0次或多次,而?
字符使其成为非贪婪。
因此,以下几行将与该正则表达式匹配:
From: [email protected]
To: !asp]<,. [email protected]
关于re语法的完整参考可在python docs找到。
关于“正确”电子邮件匹配正则表达式的示例(如练习中的),请参见此处
# Example:
import re
pattern = re.compile(r"\[(on|off)\]") # Slight optimization
print(re.search(pattern, "Mono: Playback 65 [75%] [-16.50dB] [on]"))
# Returns a Match object!
print(re.search(pattern, "Nada...:-("))
# Doesn't return anything.
# End Example
# Exercise: make a regular expression that will match an email
def test_email(your_pattern):
pattern = re.compile(your_pattern)
emails = ["[email protected]", "[email protected]", "wha.t.`1an?ug{}[email protected]"]
for email in emails:
if not re.match(pattern, email):
print("You failed to match %s" % (email))
elif not your_pattern:
print("Forgot to enter a pattern!")
else:
print("Pass")
pattern = r"" # Your pattern here!
test_email(pattern)
# Exercise: make a regular expression that will match an email
import re
def test_email(your_pattern):
pattern = re.compile(your_pattern)
emails = ["[email protected]", "[email protected]", "wha.t.`1an?ug{}[email protected]"]
for email in emails:
if not re.match(pattern, email):
print("You failed to match %s" % (email))
elif not your_pattern:
print("Forgot to enter a pattern!")
else:
print("Pass")
# Your pattern here!
pattern = r"\"?([-a-zA-Z0-9.`?{}]+@\w+\.\w+)\"?"
test_email(pattern)
test_output_contains("Pass")
success_msg("Great work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
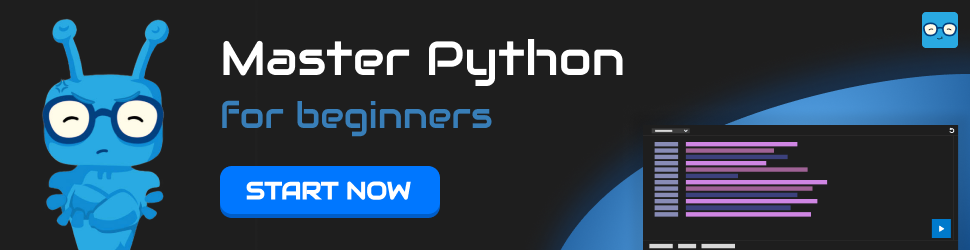