Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
פעולות בסיסיות על מחרוזות
מחרוזות הן קטעי טקסט. ניתן להגדירן כמשהו בין מירכאות:
astring = "Hello world!"
astring2 = 'Hello world!'
כפי שאתה יכול לראות, הדבר הראשון שלמדת היה הדפסת משפט פשוט. משפט זה נשמר על ידי פייתון כמחרוזת. עם זאת, במקום להדפיס מיידית את המחרוזות, נחקור את הדברים השונים שאתה יכול לעשות איתן. אתה יכול גם להשתמש במירכאות יחידות כדי להקצות מחרוזת. עם זאת, תתקל בבעיות אם הערך שברצונך להקצות מכיל בעצמו מירכאות יחידות. לדוגמה, כדי להקצות את המחרוזת בסוגריים אלו (המירכאות היחידות הן ' '), עליך להשתמש במירכאות כפולות כמו כך
astring = "Hello world!"
print("single quotes are ' '")
print(len(astring))
זה ידפיס 12, כי "Hello world!" זה 12 תווים באורך, כולל סימני פיסוק ורווחים.
astring = "Hello world!"
print(astring.index("o"))
זה ידפיס 4, כי המיקום של ההתרחשות הראשונה של האות "o" הוא 4 תווים מהתווים הראשון. שים לב שיש למעשה שני "o" במשפט - שיטה זו מכירה רק את הראשון.
אבל למה זה לא הדפיס 5? האם לא "o" הוא התו החמישי במחרוזת? כדי לפשט את הדברים, פייתון (ורוב שפות התכנות האחרות) מתחילות את המספור ב-0 במקום ב-1. לכן האינדקס של "o" הוא 4.
astring = "Hello world!"
print(astring.count("l"))
למי מכם שמשתמש בפונטים מוזרים, זהו l קטן, לא מספר אחד. זה סופר את מספר ה-l במחרוזת. לכן, זה צריך להדפיס 3.
astring = "Hello world!"
print(astring[3:7])
זה מדפיס פרוסה של המחרוזת, המתחילה באינדקס 3 ומסתיימת באינדקס 6. אבל למה 6 ולא 7? שוב, רוב שפות התכנות עושות זאת - זה מקל על ביצוע החישובים בתוך הסוגריים.
אם יש לך רק מספר אחד בסוגריים, זה ייתן לך את התו הבודד באינדקס הזה. אם תשמיט את המספר הראשון אך תשאיר את הנקודתיים, זה ייתן לך פרוסה מההתחלה עד למספר שהשארת. אם תשמיט את המספר השני, זה ייתן לך פרוסה מהמספר הראשון עד הסוף.
אתה יכול אפילו להכניס מספרים שליליים בתוך הסוגריים. הם דרך קלה להתחיל בסוף המחרוזת במקום ההתחלה. בדרך זו, -3 פירושו "התו השלישי מהסוף".
astring = "Hello world!"
print(astring[3:7:2])
זה מדפיס את התווים של המחרוזת מ-3 עד 7 תוך דילוג על תו אחד. זו תחביר פרוסה מורחב. הצורה הכללית היא [start:stop:step].
astring = "Hello world!"
print(astring[3:7])
print(astring[3:7:1])
שים לב ששניהם מייצרים את אותה תוצאה.
אין פונקציה כמו strrev ב-C להפוך מחרוזת. אבל באמצעות סוג תחביר הפרוסה שהוזכר למעלה תוכל בקלות להפוך מחרוזת כך
astring = "Hello world!"
print(astring[::-1])
זה
astring = "Hello world!"
print(astring.upper())
print(astring.lower())
אלה יוצרים מחרוזת חדשה עם כל האותיות שהומרו לאותיות גדולות וקטנות, בהתאמה.
astring = "Hello world!"
print(astring.startswith("Hello"))
print(astring.endswith("asdfasdfasdf"))
זה משמש לקביעת האם המחרוזת מתחילה במשהו או מסתיימת במשהו, בהתאמה. הראשון ידפיס True, שכן המחרוזת מתחילה ב-"Hello". השני ידפיס False, שכן המחרוזת בהחלט אינה מסתיימת ב-"asdfasdfasdf".
astring = "Hello world!"
afewwords = astring.split(" ")
זה מפרק את המחרוזת למספר מחרוזות שמקובצות יחד ברשימה. מאחר ודוגמה זו מפרידה ברווח, הפריט הראשון ברשימה יהיה "Hello", והשני יהיה "world!".
תרגיל
נסה לתקן את הקוד כדי להדפיס את המידע הנכון על ידי שינוי המחרוזת.
s = "Hey there! what should this string be?"
# Length should be 20
print("Length of s = %d" % len(s))
# First occurrence of "a" should be at index 8
print("The first occurrence of the letter a = %d" % s.index("a"))
# Number of a's should be 2
print("a occurs %d times" % s.count("a"))
# Slicing the string into bits
print("The first five characters are '%s'" % s[:5]) # Start to 5
print("The next five characters are '%s'" % s[5:10]) # 5 to 10
print("The thirteenth character is '%s'" % s[12]) # Just number 12
print("The characters with odd index are '%s'" %s[1::2]) #(0-based indexing)
print("The last five characters are '%s'" % s[-5:]) # 5th-from-last to end
# Convert everything to uppercase
print("String in uppercase: %s" % s.upper())
# Convert everything to lowercase
print("String in lowercase: %s" % s.lower())
# Check how a string starts
if s.startswith("Str"):
print("String starts with 'Str'. Good!")
# Check how a string ends
if s.endswith("ome!"):
print("String ends with 'ome!'. Good!")
# Split the string into three separate strings,
# each containing only a word
print("Split the words of the string: %s" % s.split(" "))
s = "Strings are awesome!"
# Length should be 20
print("Length of s = %d" % len(s))
# First occurrence of "a" should be at index 8
print("The first occurrence of the letter a = %d" % s.index("a"))
# Number of a's should be 2
print("a occurs %d times" % s.count("a"))
# Slicing the string into bits
print("The first five characters are '%s'" % s[:5]) # Start to 5
print("The next five characters are '%s'" % s[5:10]) # 5 to 10
print("The thirteenth character is '%s'" % s[12]) # Just number 12
print("The characters with odd index are '%s'" %s[1::2]) #(0-based indexing)
print("The last five characters are '%s'" % s[-5:]) # 5th-from-last to end
# Convert everything to uppercase
print("String in uppercase: %s" % s.upper())
# Convert everything to lowercase
print("String in lowercase: %s" % s.lower())
# Check how a string starts
if s.startswith("Str"):
print("String starts with 'Str'. Good!")
# Check how a string ends
if s.endswith("ome!"):
print("String ends with 'ome!'. Good!")
# Split the string into three separate strings,
# each containing only a word
print("Split the words of the string: %s" % s.split(" "))
test_object("s", incorrect_msg="Make sure you change the string assigned to `s` to match the exercise instructions.")
success_msg("Great work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
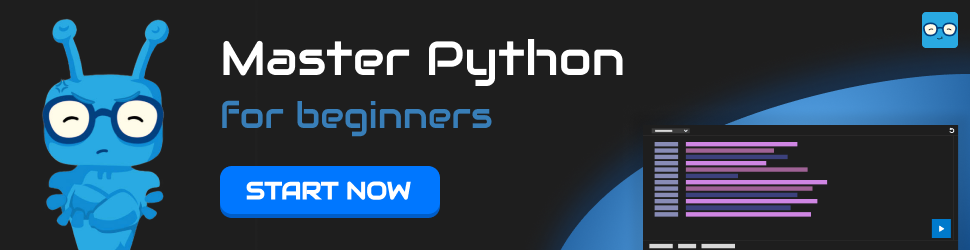