Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
תנאים
Python משתמשת בלוגיקה בוליאנית להערכת תנאים. הערכים הבוליאניים True ו-False מוחזרים כאשר ביטוי מושווה או מוערך. לדוגמה:
x = 2 print(x == 2) # מדפיס True print(x == 3) # מדפיס False print(x < 3) # מדפיס True ```
שימו לב שהשמת משתנה נעשית באמצעות סימן שווה בודד "=", בעוד השוואה בין שני משתנים נעשית באמצעות סימן שווה כפול "==". האופרטור "לא שווה" מסומן כ- "!=".
אופרטורים בוליאניים
האופרטורים הבוליאניים "and" ו-"or" מאפשרים לבנות ביטויים בוליאניים מורכבים, לדוגמה:
name = "John" age = 23 if name == "John" and age == 23: print("Your name is John, and you are also 23 years old.")
if name == "John" or name == "Rick": print("Your name is either John or Rick.") ```
אופרטור ה-"in"
ניתן להשתמש באופרטור ה-"in" כדי לבדוק אם אובייקט מסוים קיים בתוך אובייקט ניתן לחקר, כמו רשימה:
name = "John" if name in ["John", "Rick"]: print("Your name is either John or Rick.") ```
Python משתמשת בכניסות כדי להגדיר בלוקים של קוד, במקום סוגריים. הכניסה הסטנדרטית ב-Python היא 4 רווחים, למרות שטאבים וכל גודל רווח אחר יעבוד, כל עוד זה עקבי. שימו לב שבלוקים של קוד לא מצריכים שום סיום.
הנה דוגמה לשימוש במשפט ה-"if" של Python עם בלוקים של קוד:
statement = False
another_statement = True
if statement is True:
# עשה משהו
pass
elif another_statement is True: # אחרת אם
# עשה משהו אחר
pass
else:
# עשה דבר אחר
pass
לדוגמה:
x = 2
if x == 2:
print("x equals two!")
else:
print("x does not equal to two.")
משפט יוערך כנכון אם אחד מהבאים נכון: 1. משתנה בוליאני "True" ניתן, או מחושב באמצעות ביטוי, כמו השוואה אריתמטית. 2. אובייקט שאינו נחשב "ריק" מועבר.
הנה כמה דוגמאות לאובייקטים הנחשבים כריקים: 1. מחרוזת ריקה: "" 2. רשימה ריקה: [] 3. המספר אפס: 0 4. משתנה בוליאני שגוי: False
אופרטור 'is'
שלא כמו אופרטור השווה הכפול "==", האופרטור "is" לא מתאים את הערכים של המשתנים, אלא את המופעים עצמם. לדוגמה:
x = [1,2,3]
y = [1,2,3]
print(x == y) # מדפיס True
print(x is y) # מדפיס False
אופרטור "not"
שימוש ב-"not" לפני ביטוי בוליאני הופך אותו:
python
print(not False) # מדפיס True
print((not False) == (False)) # מדפיס False
תרגיל
שנה את המשתנים בחלק הראשון, כך שכל משפט if ייפתר כ-True.
# change this code
number = 10
second_number = 10
first_array = []
second_array = [1,2,3]
if number > 15:
print("1")
if first_array:
print("2")
if len(second_array) == 2:
print("3")
if len(first_array) + len(second_array) == 5:
print("4")
if first_array and first_array[0] == 1:
print("5")
if not second_number:
print("6")
# change this code
number = 16
second_number = 0
first_array = [1,2,3]
second_array = [1,2]
if number > 15:
print("1")
if first_array:
print("2")
if len(second_array) == 2:
print("3")
if len(first_array) + len(second_array) == 5:
print("4")
if first_array and first_array[0] == 1:
print("5")
if not second_number:
print("6")
test_output_contains("1", no_output_msg= "Did you print out 1 if `number` is greater than 15?")
test_output_contains("2", no_output_msg= "Did you print out 2 if there exists a list `first_array`?")
test_output_contains("3", no_output_msg= "Did you print out 3 if the length of `second_array` is 2?")
test_output_contains("4", no_output_msg= "Did you print out 4 if len(first_array) + len(second_array) == 5?")
test_output_contains("5", no_output_msg= "Did you print out 5 if first_array and first_array[0] == 1?")
test_output_contains("6", no_output_msg= "Did you print out 6 if not second_number?")
success_msg("Great Work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
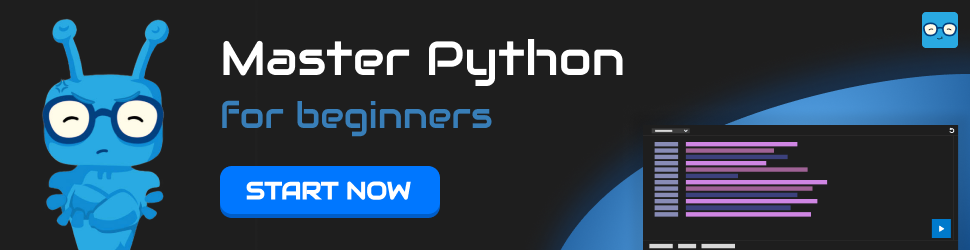