Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
基本的な文字列操作
コンテンツ: 文字列はテキストの断片です。引用符で囲まれたものが文字列として定義されます。
astring = "Hello world!" astring2 = 'Hello world!'
ご覧のとおり、最初に学んだのは、簡単な文を印刷することでした。この文はPythonによって文字列として保存されました。ただし、文字列をすぐに印刷するのではなく、それらに対してできるさまざまな操作を探っていきます。文字列を割り当てるのにシングルクォートも使用できます。ただし、割り当てる値自体にシングルクォートが含まれている場合は問題が発生します。例として、括弧内の文字列(シングルクォートが ' ')を割り当てるには、ダブルクォートのみを使用する必要があります、このように
astring = "Hello world!" print("single quotes are ' '")
print(len(astring))
これは12を印刷します。なぜなら、"Hello world!"は句読点やスペースを含めて12文字だからです。
astring = "Hello world!" print(astring.index("o"))
これは4を印刷します。なぜなら、"o"が最初に登場する場所は先頭から4文字の距離にあるからです。このフレーズには実際には2つのoがありますが、このメソッドは最初のものだけを認識します。
でもなぜ5ではないのでしょうか?文字列の中で"o"は5番目の文字ではないでしょうか?もっと単純にするために、Python(およびほとんどの他のプログラミング言語)は0からスタートします。そのため、"o"のインデックスは4です。
astring = "Hello world!" print(astring.count("l"))
これは小文字のLであり、一の数字ではありません。これは文字列内のlの数を数えます。したがって、3を印刷します。
astring = "Hello world!" print(astring[3:7])
これは文字列の一部を印刷します。インデックス3から始まり、インデックス6で終わります。でもなぜ6なのか、7ではないのでしょうか?多くのプログラミング言語がこの方法を取っており、その方が括弧内での計算が簡単になります。
括弧内に数字を1つだけ記入すると、そのインデックスの単一の文字が得られます。最初の番号を省略してコロンを残すと、最初から残された番号までのスライスが得られます。2番目の番号を省略すると、最初の番号から最後までのスライスが得られます。
括弧内に負の数字を入れることもできます。これにより、文字列の終わりから逆にスタートすることが簡単になります。この方法では、-3が「終わりから3番目の文字」を意味します。
astring = "Hello world!" print(astring[3:7:2])
これはインデックス3から7までの文字を、1文字飛ばしで印刷します。これは拡張されたスライス構文です。一般的な形式は[start:stop:step]です。
astring = "Hello world!" print(astring[3:7]) print(astring[3:7:1])
どちらも同じ出力を生成することに注意してください。
C言語のstrrevのように文字列を逆転する関数はありませんが、上述のスライス構文を使えばこのように容易に文字列を逆転できます。
astring = "Hello world!" print(astring[::-1])
このように
astring = "Hello world!" print(astring.upper()) print(astring.lower())
これにより、すべての文字がそれぞれ大文字と小文字に変換された新しい文字列が作成されます。
astring = "Hello world!" print(astring.startswith("Hello")) print(astring.endswith("asdfasdfasdf"))
これは文字列が何かで始まるか、または何かで終わるかを判断するために使用されます。最初のものはTrueを印刷します。なぜなら、文字列は"Hello"で始まるからです。2番目のものはFalseを印刷します。なぜなら、文字列は"asdfasdfasdf"で確かに終わらないからです。
astring = "Hello world!" afewwords = astring.split(" ")
これは文字列をリストとしてまとめられた一連の文字列に分割します。この例ではスペースで分割されるため、リストの最初の項目は"Hello"で、2番目は"world!"になります。
演習
文字列を変更して、正しい情報を印刷するようにコードを修正してみてください。
s = "Hey there! what should this string be?"
# Length should be 20
print("Length of s = %d" % len(s))
# First occurrence of "a" should be at index 8
print("The first occurrence of the letter a = %d" % s.index("a"))
# Number of a's should be 2
print("a occurs %d times" % s.count("a"))
# Slicing the string into bits
print("The first five characters are '%s'" % s[:5]) # Start to 5
print("The next five characters are '%s'" % s[5:10]) # 5 to 10
print("The thirteenth character is '%s'" % s[12]) # Just number 12
print("The characters with odd index are '%s'" %s[1::2]) #(0-based indexing)
print("The last five characters are '%s'" % s[-5:]) # 5th-from-last to end
# Convert everything to uppercase
print("String in uppercase: %s" % s.upper())
# Convert everything to lowercase
print("String in lowercase: %s" % s.lower())
# Check how a string starts
if s.startswith("Str"):
print("String starts with 'Str'. Good!")
# Check how a string ends
if s.endswith("ome!"):
print("String ends with 'ome!'. Good!")
# Split the string into three separate strings,
# each containing only a word
print("Split the words of the string: %s" % s.split(" "))
s = "Strings are awesome!"
# Length should be 20
print("Length of s = %d" % len(s))
# First occurrence of "a" should be at index 8
print("The first occurrence of the letter a = %d" % s.index("a"))
# Number of a's should be 2
print("a occurs %d times" % s.count("a"))
# Slicing the string into bits
print("The first five characters are '%s'" % s[:5]) # Start to 5
print("The next five characters are '%s'" % s[5:10]) # 5 to 10
print("The thirteenth character is '%s'" % s[12]) # Just number 12
print("The characters with odd index are '%s'" %s[1::2]) #(0-based indexing)
print("The last five characters are '%s'" % s[-5:]) # 5th-from-last to end
# Convert everything to uppercase
print("String in uppercase: %s" % s.upper())
# Convert everything to lowercase
print("String in lowercase: %s" % s.lower())
# Check how a string starts
if s.startswith("Str"):
print("String starts with 'Str'. Good!")
# Check how a string ends
if s.endswith("ome!"):
print("String ends with 'ome!'. Good!")
# Split the string into three separate strings,
# each containing only a word
print("Split the words of the string: %s" % s.split(" "))
test_object("s", incorrect_msg="Make sure you change the string assigned to `s` to match the exercise instructions.")
success_msg("Great work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
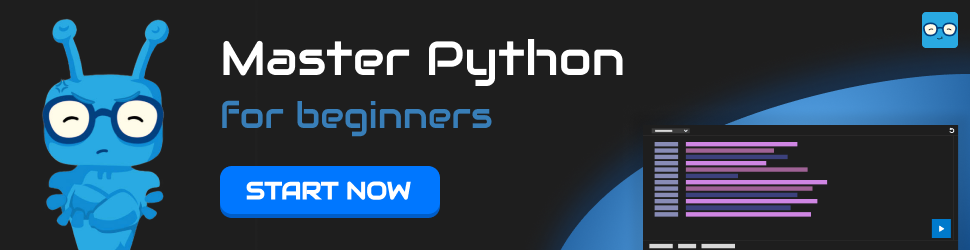