Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
基本演算子
このセクションでは、Pythonの基本的な演算子の使い方を説明します。
算術演算子
他のプログラミング言語と同様に、加算、減算、乗算、除算の演算子は数値に使用できます。
number = 1 + 2 * 3 / 4.0
print(number)
答えが何になるか予測してみてください。Pythonは算術の優先順位に従いますか?
別の演算子として、割り算の剰余を返す剰余演算子(%)があります。 被除数 % 除数 = 剰余。
remainder = 11 % 3
print(remainder)
乗算記号を2つ使用すると、べき乗関係を表します。
squared = 7 ** 2
cubed = 2 ** 3
print(squared)
print(cubed)
文字列での演算子の使用
Pythonは文字列の結合に加算演算子をサポートしています:
helloworld = "hello" + " " + "world"
print(helloworld)
また、Pythonでは文字列を乗算して繰り返しのシーケンスを含む文字列を生成することもサポートしています:
lotsofhellos = "hello" * 10
print(lotsofhellos)
リストでの演算子の使用
リストは加算演算子で結合することができます:
even_numbers = [2,4,6,8]
odd_numbers = [1,3,5,7]
all_numbers = odd_numbers + even_numbers
print(all_numbers)
同様に、文字列と同様に、Pythonは乗算演算子を使用して繰り返しのシーケンスを含む新しいリストを作成することもできます:
print([1,2,3] * 3)
演習
この演習の目標は、x_list
とy_list
という2つのリストを作成することです。それぞれのリストには変数x
とy
が10個含まれています。さらに、作成した2つのリストを結合して、変数x
とy
がそれぞれ10回含まれているbig_list
を作成することです。
x = object()
y = object()
# TODO: change this code
x_list = [x]
y_list = [y]
big_list = []
print("x_list contains %d objects" % len(x_list))
print("y_list contains %d objects" % len(y_list))
print("big_list contains %d objects" % len(big_list))
# testing code
if x_list.count(x) == 10 and y_list.count(y) == 10:
print("Almost there...")
if big_list.count(x) == 10 and big_list.count(y) == 10:
print("Great!")
x = object()
y = object()
# TODO: change this code
x_list = [x] * 10
y_list = [y] * 10
big_list = x_list + y_list
print("x_list contains %d objects" % len(x_list))
print("y_list contains %d objects" % len(y_list))
print("big_list contains %d objects" % len(big_list))
# testing code
if x_list.count(x) == 10 and y_list.count(y) == 10:
print("Almost there...")
if big_list.count(x) == 10 and big_list.count(y) == 10:
print("Great!")
Ex().check_object('x_list').has_equal_value(expr_code = 'len(x_list)')
Ex().check_object('y_list').has_equal_value(expr_code = 'len(y_list)')
Ex().check_object('big_list').has_equal_value(expr_code = 'len(big_list)')
success_msg('Good work!')
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
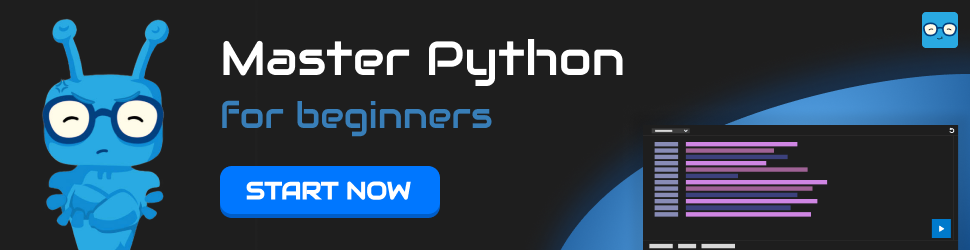