Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
ジェネレーター
ジェネレーターは実装が非常に簡単ですが、少し理解しにくい場合があります。
ジェネレーターはイテレーターを作成するために使用されますが、異なるアプローチを取ります。ジェネレーターは、特別な方法で、1回に1つずつ反復可能なアイテムのセットを返す単純な関数です。
for文を使用してアイテムのセットを反復処理する際に、ジェネレーターが実行されます。ジェネレーターの関数コードが「yield」文に達すると、ジェネレーターは実行をforループに戻し、セットから新しい値を返します。ジェネレーター関数は、望むだけ多くの値(おそらく無限に)を生成し、順番に各値をyieldすることができます。
次に、7つのランダムな整数を返すジェネレーターの簡単な例があります:
import random
def lottery():
# returns 6 numbers between 1 and 40
for i in range(6):
yield random.randint(1, 40)
# returns a 7th number between 1 and 15
yield random.randint(1, 15)
for random_number in lottery():
print("And the next number is... %d!" %(random_number))
この関数は、ランダムな数字をどのように生成するかを独自に判断し、各yield文を1つずつ実行し、その間にメインのforループに実行を戻して一時停止します。
演習
フィボナッチ数列を返すジェネレーター関数を書いてください。それらは次の式を使用して計算されます:数列の最初の2つの数は常に1に等しく、返される各連続する数は直前の2つの数の合計です。 ヒント: ジェネレーター関数で2つの変数だけを使用することができますか?代入は同時に行うことができることを思い出してください。このコード
a = 1
b = 2
a, b = b, a
print(a, b)
はaとbの値を同時に交換します。
# fill in this function
def fib():
pass #this is a null statement which does nothing when executed, useful as a placeholder.
# testing code
import types
if type(fib()) == types.GeneratorType:
print("Good, The fib function is a generator.")
counter = 0
for n in fib():
print(n)
counter += 1
if counter == 10:
break
# fill in this function
def fib():
a, b = 1, 1
while 1:
yield a
a, b = b, a + b
# testing code
import types
if type(fib()) == types.GeneratorType:
print("Good, The fib function is a generator.")
counter = 0
for n in fib():
print(n)
counter += 1
if counter == 10:
break
test_output_contains("Good, The fib function is a generator.")
success_msg('Good work!')
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
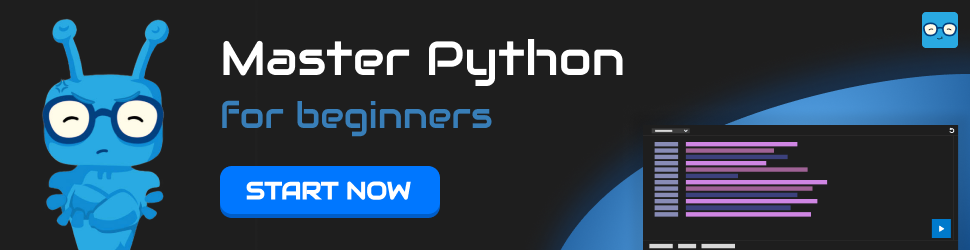