Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
ループ
Pythonには2種類のループがあります。forとwhileです。
The "for" loop
forループは、指定されたシーケンスを反復します。以下はその例です:
primes = [2, 3, 5, 7]
for prime in primes:
print(prime)
forループは、"range"や"xrange"関数を使って数のシーケンスを反復することができます。rangeとxrangeの違いは、range関数が指定された範囲の数で新しいリストを返すのに対し、xrangeはイテレータを返します。これはより効率的です。(Python 3ではrange関数が使われ、xrangeのように動作します)。range関数は0から始まることに注意してください。
# 0,1,2,3,4を出力します
for x in range(5):
print(x)
# 3,4,5を出力します
for x in range(3, 6):
print(x)
# 3,5,7を出力します
for x in range(3, 8, 2):
print(x)
"while"ループ
whileループは、指定されたブール条件が満たされている限り繰り返されます。例えば:
# 0,1,2,3,4を出力します
count = 0
while count < 5:
print(count)
count += 1 # これはcount = count + 1と同じです
"break"と"continue"ステートメント
breakは、forループやwhileループを終了するために使われます。一方で、continueは現在のブロックをスキップし、「for」または「while」文に戻ります。以下はいくつかの例です:
# 0,1,2,3,4を出力します
count = 0
while True:
print(count)
count += 1
if count >= 5:
break
# 奇数のみを出力します - 1,3,5,7,9
for x in range(10):
# xが偶数かどうかをチェックします
if x % 2 == 0:
continue
print(x)
ループに"else"節を使用できますか?
C、CPPのような言語とは異なり、ループにelseを使用することができます。「for」または「while」文のループ条件が失敗した場合、「else」内のコードが実行されます。forループ内でbreakステートメントが実行された場合、「else」部分はスキップされます。continueステートメントがあっても「else」部分が実行されることに注意してください。
以下はいくつかの例です:
# 0,1,2,3,4を出力し、その後に"count value reached 5"と出力します
count=0
while(count<5):
print(count)
count +=1
else:
print("count value reached %d" %(count))
# 1,2,3,4を出力します
for i in range(1, 10):
if(i%5==0):
break
print(i)
else:
print("これは印刷されません。なぜならforループは条件が失敗したためではなくbreakによって終了されたためです")
Exercise
numbersリストのすべての偶数を、それらが受け取られた順序でループして出力してください。237の後に続く数字は出力しないでください。
numbers = [
951, 402, 984, 651, 360, 69, 408, 319, 601, 485, 980, 507, 725, 547, 544,
615, 83, 165, 141, 501, 263, 617, 865, 575, 219, 390, 984, 592, 236, 105, 942, 941,
386, 462, 47, 418, 907, 344, 236, 375, 823, 566, 597, 978, 328, 615, 953, 345,
399, 162, 758, 219, 918, 237, 412, 566, 826, 248, 866, 950, 626, 949, 687, 217,
815, 67, 104, 58, 512, 24, 892, 894, 767, 553, 81, 379, 843, 831, 445, 742, 717,
958, 609, 842, 451, 688, 753, 854, 685, 93, 857, 440, 380, 126, 721, 328, 753, 470,
743, 527
]
# your code goes here
for number in numbers:
numbers = [
951, 402, 984, 651, 360, 69, 408, 319, 601, 485, 980, 507, 725, 547, 544,
615, 83, 165, 141, 501, 263, 617, 865, 575, 219, 390, 984, 592, 236, 105, 942, 941,
386, 462, 47, 418, 907, 344, 236, 375, 823, 566, 597, 978, 328, 615, 953, 345,
399, 162, 758, 219, 918, 237, 412, 566, 826, 248, 866, 950, 626, 949, 687, 217,
815, 67, 104, 58, 512, 24, 892, 894, 767, 553, 81, 379, 843, 831, 445, 742, 717,
958, 609, 842, 451, 688, 753, 854, 685, 93, 857, 440, 380, 126, 721, 328, 753, 470,
743, 527
]
# your code goes here
for number in numbers:
if number == 237:
break
if number % 2 == 1:
continue
print(number)
test_object("number", undefined_msg="Define a object `number` using the code from the tutorial to print just the desired numbers from the exercise description.",incorrect_msg="Your `number` object is not correct, You should use an `if` statement and a `break` statement to accomplish your goal.")
success_msg("Great work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
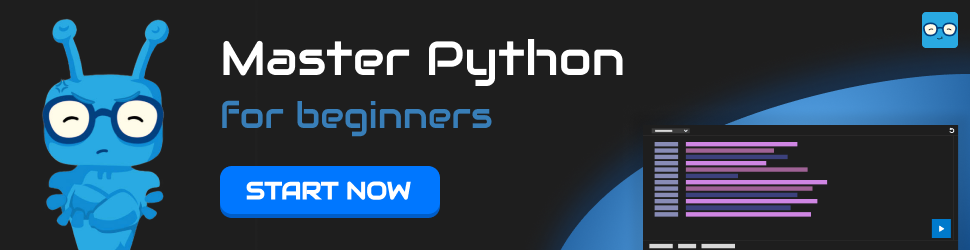