Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
正規表現
Regular Expressions
正規表現(時々regexp、regex、またはreと略される)は、テキスト内のパターンを一致させるためのツールです。Pythonでは、reモジュールがあります。正規表現の用途は多岐にわたりますが、かなり複雑です。そのため、あるタスクに正規表現を使用しようと考える際には、他の選択肢について考え、最後の手段として正規表現を考えるようにしてください。
例として、r"^(From|To|Cc).*[email protected]"
という正規表現を見てみましょう。説明としては、キャレット記号 ^
は行の先頭のテキストに一致します。その後のグループ、 (From|To|Cc)
という部分は、行がパイプ |
で区切られた単語のいずれかで始まることを意味します。これはOR演算子と呼ばれ、グループ内のいずれかの単語で行が始まる場合に正規表現は一致します。.*?
は、改行 \n
文字を除く任意の数の文字に非貪欲に一致します。非貪欲とは、できるだけ少ない繰り返しに一致することを意味します。 .
文字は改行以外の任意の文字を意味し、 *
は0回以上の繰り返しを意味し、 ?
はそれを非貪欲にします。
そのため、次の行はこの正規表現に一致します:
From: [email protected]
To: !asp]<,. [email protected]
正規表現の構文の完全なリファレンスは、python docsで参照できます。
"正しい"メールアドレスを一致させる正規表現の例(演習にあるもののような)として、こちらを参照してください。
# Example:
import re
pattern = re.compile(r"\[(on|off)\]") # Slight optimization
print(re.search(pattern, "Mono: Playback 65 [75%] [-16.50dB] [on]"))
# Returns a Match object!
print(re.search(pattern, "Nada...:-("))
# Doesn't return anything.
# End Example
# Exercise: make a regular expression that will match an email
def test_email(your_pattern):
pattern = re.compile(your_pattern)
emails = ["[email protected]", "[email protected]", "wha.t.`1an?ug{}[email protected]"]
for email in emails:
if not re.match(pattern, email):
print("You failed to match %s" % (email))
elif not your_pattern:
print("Forgot to enter a pattern!")
else:
print("Pass")
pattern = r"" # Your pattern here!
test_email(pattern)
# Exercise: make a regular expression that will match an email
import re
def test_email(your_pattern):
pattern = re.compile(your_pattern)
emails = ["[email protected]", "[email protected]", "wha.t.`1an?ug{}[email protected]"]
for email in emails:
if not re.match(pattern, email):
print("You failed to match %s" % (email))
elif not your_pattern:
print("Forgot to enter a pattern!")
else:
print("Pass")
# Your pattern here!
pattern = r"\"?([-a-zA-Z0-9.`?{}]+@\w+\.\w+)\"?"
test_email(pattern)
test_output_contains("Pass")
success_msg("Great work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
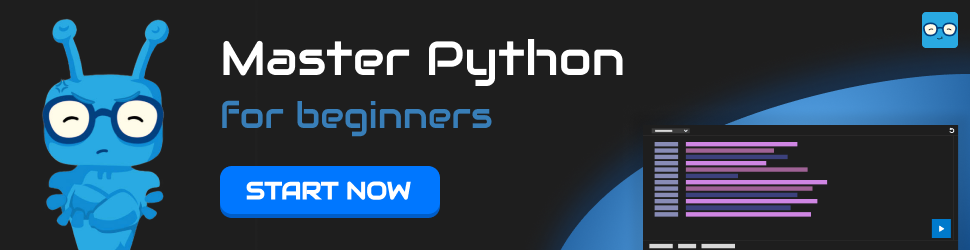