Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Keadaan
Python menggunakan logik boolean untuk menilai syarat. Nilai boolean True dan False dikembalikan apabila suatu ekspresi dibandingkan atau dinilai. Sebagai contoh:
x = 2
print(x == 2) # mencetak True
print(x == 3) # mencetak False
print(x < 3) # mencetak True
Perhatikan bahawa penugasan pembolehubah dilakukan menggunakan satu operator sama "=" manakala perbandingan antara dua pembolehubah dilakukan menggunakan operator sama berganda "==". Operator "tidak sama" ditandakan sebagai "!=".
Operator boolean
Operator boolean "and" dan "or" membolehkan pembangunan ekspresi boolean yang kompleks, sebagai contoh:
name = "John"
age = 23
if name == "John" and age == 23:
print("Your name is John, and you are also 23 years old.")
if name == "John" or name == "Rick":
print("Your name is either John or Rick.")
Operator "in"
Operator "in" boleh digunakan untuk memeriksa jika suatu objek yang ditentukan wujud dalam bekas objek iterable, seperti senarai:
name = "John"
if name in ["John", "Rick"]:
print("Your name is either John or Rick.")
Python menggunakan indentasi untuk mentakrifkan blok kod, bukannya kurungan. Indentasi Python standard adalah 4 ruang, walaupun tab dan saiz ruang lain akan berfungsi, asalkan konsisten. Perhatikan bahawa blok kod tidak memerlukan sebarang penamatan.
Ini adalah contoh penggunaan penyataan "if" dalam Python menggunakan blok kod:
statement = False
another_statement = True
if statement is True:
# melakukan sesuatu
pass
elif another_statement is True: # else if
# melakukan perkara lain
pass
else:
# melakukan perkara lain lagi
pass
Sebagai contoh:
x = 2
if x == 2:
print("x equals two!")
else:
print("x does not equal to two.")
Satu pernyataan dinilai sebagai benar jika salah satu daripada yang berikut adalah betul: 1. Pembolehubah boolean "True" diberikan, atau dikira menggunakan ekspresi, seperti perbandingan aritmetik. 2. Sebuah objek yang tidak dianggap "kosong" dilewati.
Berikut adalah beberapa contoh objek yang dianggap kosong: 1. String kosong: "" 2. Senarai kosong: [] 3. Nombor sifar: 0 4. Pembolehubah boolean palsu: False
Operator 'is'
Tidak seperti operator sama berganda "==", operator "is" tidak menyamai nilai pembolehubah tetapi contoh mereka sendiri. Sebagai contoh:
x = [1,2,3]
y = [1,2,3]
print(x == y) # Mencetak True
print(x is y) # Mencetak False
Operator "not"
Menggunakan "not" sebelum ekspresi boolean membalikkannya:
print(not False) # Mencetak True
print((not False) == (False)) # Mencetak False
Exercise
Ubah pembolehubah dalam bahagian pertama, supaya setiap penyataan if diselesaikan sebagai True.
# change this code
number = 10
second_number = 10
first_array = []
second_array = [1,2,3]
if number > 15:
print("1")
if first_array:
print("2")
if len(second_array) == 2:
print("3")
if len(first_array) + len(second_array) == 5:
print("4")
if first_array and first_array[0] == 1:
print("5")
if not second_number:
print("6")
# change this code
number = 16
second_number = 0
first_array = [1,2,3]
second_array = [1,2]
if number > 15:
print("1")
if first_array:
print("2")
if len(second_array) == 2:
print("3")
if len(first_array) + len(second_array) == 5:
print("4")
if first_array and first_array[0] == 1:
print("5")
if not second_number:
print("6")
test_output_contains("1", no_output_msg= "Did you print out 1 if `number` is greater than 15?")
test_output_contains("2", no_output_msg= "Did you print out 2 if there exists a list `first_array`?")
test_output_contains("3", no_output_msg= "Did you print out 3 if the length of `second_array` is 2?")
test_output_contains("4", no_output_msg= "Did you print out 4 if len(first_array) + len(second_array) == 5?")
test_output_contains("5", no_output_msg= "Did you print out 5 if first_array and first_array[0] == 1?")
test_output_contains("6", no_output_msg= "Did you print out 6 if not second_number?")
success_msg("Great Work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
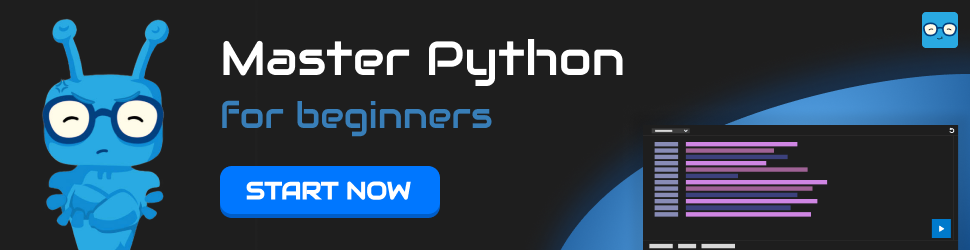