Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Pembolehubah dan Jenis
Python sepenuhnya berorientasikan objek, dan tidak "diketik secara statik". Anda tidak perlu mengisytiharkan pembolehubah sebelum menggunakannya, atau mengisytiharkan jenisnya. Setiap pembolehubah dalam Python adalah objek.
Tutorial ini akan merangkumi beberapa jenis asas pembolehubah.
Nombor
Python menyokong dua jenis nombor - integer (nombor bulat) dan nombor titik terapung (nombor perpuluhan). (Ia juga menyokong nombor kompleks, yang tidak akan dijelaskan dalam tutorial ini).
Untuk mendefinisikan integer, gunakan sintaks berikut:
myint = 7
print(myint)
Untuk mendefinisikan nombor titik terapung, anda boleh menggunakan salah satu notasi berikut:
myfloat = 7.0
print(myfloat)
myfloat = float(7)
print(myfloat)
Rentetan
Rentetan didefinisikan sama ada dengan tanda petikan tunggal atau tanda petikan dua.
mystring = 'hello'
print(mystring)
mystring = "hello"
print(mystring)
Perbezaan antara keduanya adalah bahawa menggunakan tanda petikan dua memudahkan untuk memasukkan apostrof (walaupun ini akan menamatkan rentetan jika menggunakan tanda petikan tunggal)
mystring = "Don't worry about apostrophes"
print(mystring)
Terdapat variasi tambahan dalam mendefinisikan rentetan yang memudahkan untuk memasukkan perkara seperti carriage return, garis miring terbalik dan aksara Unicode. Ini berada di luar skop tutorial ini, tetapi dibahas dalam dokumentasi Python.
Pengoperasi mudah boleh dieksekusi pada nombor dan rentetan:
one = 1
two = 2
three = one + two
print(three)
hello = "hello"
world = "world"
helloworld = hello + " " + world
print(helloworld)
Pengagihan boleh dilakukan pada lebih daripada satu pembolehubah "serentak" pada baris yang sama seperti ini
a, b = 3, 4
print(a, b)
Pencampuran pengoperasi antara nombor dan rentetan tidak disokong:
# Ini tidak akan berfungsi!
one = 1
two = 2
hello = "hello"
print(one + two + hello)
Latihan
Matlamat latihan ini adalah untuk membuat rentetan, integer, dan nombor titik terapung. Rentetan itu sepatutnya dinamakan mystring
dan harus mengandungi perkataan "hello". Nombor titik terapung sepatutnya dinamakan myfloat
dan harus mengandungi nombor 10.0, dan integer sepatutnya dinamakan myint
dan harus mengandungi nombor 20.
# change this code
mystring = None
myfloat = None
myint = None
# testing code
if mystring == "hello":
print("String: %s" % mystring)
if isinstance(myfloat, float) and myfloat == 10.0:
print("Float: %f" % myfloat)
if isinstance(myint, int) and myint == 20:
print("Integer: %d" % myint)
# change this code
mystring = "hello"
myfloat = 10.0
myint = 20
# testing code
if mystring == "hello":
print("String: %s" % mystring)
if isinstance(myfloat, float) and myfloat == 10.0:
print("Float: %f" % myfloat)
if isinstance(myint, int) and myint == 20:
print("Integer: %d" % myint)
test_object('mystring', incorrect_msg="Don't forget to change `mystring` to the correct value from the exercise description.")
test_object('myfloat', incorrect_msg="Don't forget to change `myfloat` to the correct value from the exercise description.")
test_object('myint', incorrect_msg="Don't forget to change `myint` to the correct value from the exercise description.")
test_output_contains("String: hello",no_output_msg= "Make sure your string matches exactly to the exercise desciption.")
test_output_contains("Float: 10.000000",no_output_msg= "Make sure your float matches exactly to the exercise desciption.")
test_output_contains("Integer: 20",no_output_msg= "Make sure your integer matches exactly to the exercise desciption.")
success_msg("Great job!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
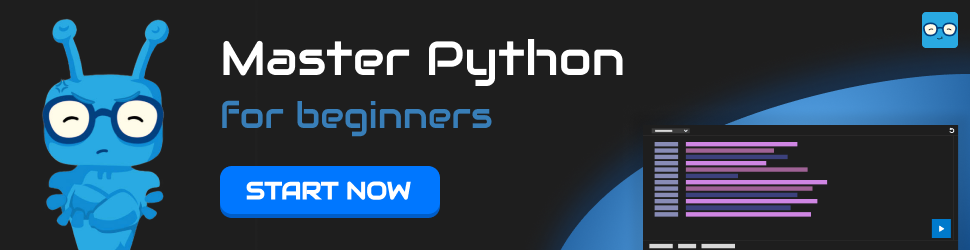