Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Toán Tử Cơ Bản
Certainly! Here's the translated content following your specified guidelines:
Nội dung phần này giải thích cách sử dụng các toán tử cơ bản trong Python.
Arithmetic Operators
Giống như bất kỳ ngôn ngữ lập trình nào khác, các toán tử cộng, trừ, nhân và chia có thể được sử dụng với số.
number = 1 + 2 * 3 / 4.0
print(number)
Hãy thử dự đoán kết quả sẽ là gì. Python có tuân theo thứ tự thực hiện không?
Một toán tử khác có sẵn là toán tử modulo (%) để trả về phần dư của phép chia nguyên. Số bị chia % số chia = phần dư.
remainder = 11 % 3
print(remainder)
Sử dụng hai ký hiệu nhân thể hiện mũ.
squared = 7 ** 2
cubed = 2 ** 3
print(squared)
print(cubed)
Using Operators with Strings
Python hỗ trợ nối chuỗi bằng toán tử cộng:
helloworld = "hello" + " " + "world"
print(helloworld)
Python cũng hỗ trợ nhân chuỗi để tạo ra chuỗi với một dãy lặp lại:
lotsofhellos = "hello" * 10
print(lotsofhellos)
Using Operators with Lists
Danh sách có thể được nối với nhau bằng toán tử cộng:
even_numbers = [2,4,6,8]
odd_numbers = [1,3,5,7]
all_numbers = odd_numbers + even_numbers
print(all_numbers)
Giống như với chuỗi, Python hỗ trợ tạo danh sách mới với một dãy lặp lại bằng toán tử nhân:
print([1,2,3] * 3)
Exercise
Mục tiêu của bài tập này là tạo hai danh sách gọi là x_list
và y_list
,
chứa 10 phần tử là biến x
và y
tương ứng.
Bạn cũng cần tạo một danh sách gọi là big_list
, chứa
các biến x
và y
, mỗi biến 10 lần, bằng cách nối hai danh sách mà bạn đã tạo.
x = object()
y = object()
# TODO: change this code
x_list = [x]
y_list = [y]
big_list = []
print("x_list contains %d objects" % len(x_list))
print("y_list contains %d objects" % len(y_list))
print("big_list contains %d objects" % len(big_list))
# testing code
if x_list.count(x) == 10 and y_list.count(y) == 10:
print("Almost there...")
if big_list.count(x) == 10 and big_list.count(y) == 10:
print("Great!")
x = object()
y = object()
# TODO: change this code
x_list = [x] * 10
y_list = [y] * 10
big_list = x_list + y_list
print("x_list contains %d objects" % len(x_list))
print("y_list contains %d objects" % len(y_list))
print("big_list contains %d objects" % len(big_list))
# testing code
if x_list.count(x) == 10 and y_list.count(y) == 10:
print("Almost there...")
if big_list.count(x) == 10 and big_list.count(y) == 10:
print("Great!")
Ex().check_object('x_list').has_equal_value(expr_code = 'len(x_list)')
Ex().check_object('y_list').has_equal_value(expr_code = 'len(y_list)')
Ex().check_object('big_list').has_equal_value(expr_code = 'len(big_list)')
success_msg('Good work!')
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
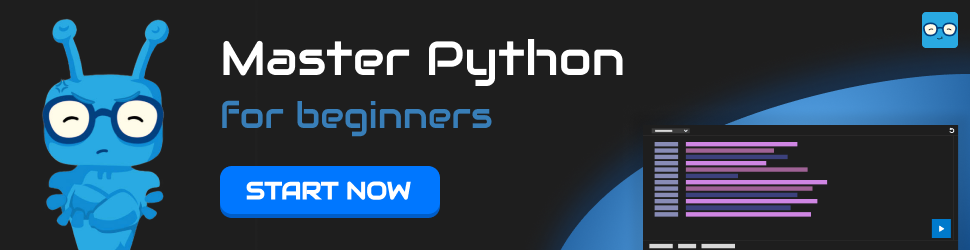