Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Chức năng
Chức năng là gì?
Các chức năng giúp chúng ta chia nhỏ mã nguồn thành các khối có ích, giúp sắp xếp mã nguồn, làm cho mã dễ đọc hơn, tái sử dụng nó và tiết kiệm thời gian. Ngoài ra, các chức năng là cách quan trọng để định nghĩa các giao diện để lập trình viên có thể chia sẻ mã của họ.
Làm thế nào để viết hàm trong Python?
Như chúng ta đã thấy trong các hướng dẫn trước, Python sử dụng các khối mã.
Một khối là một khu vực mã được viết theo định dạng:
block_head:
1st block line
2nd block line
...
Nơi một dòng khối là mã Python khác (thậm chí một khối khác), và đầu khối có định dạng sau: block_keyword block_name(argument1,argument2, ...) Các từ khóa khối bạn đã biết là "if", "for", và "while".
Hàm trong python được định nghĩa bằng từ khóa khối "def", theo sau là tên của hàm như là tên của khối. Ví dụ:
def my_function():
print("Hello From My Function!")
Các hàm cũng có thể nhận các đối số (các biến được truyền từ người gọi đến hàm). Ví dụ:
def my_function_with_args(username, greeting):
print("Hello, %s , From My Function!, I wish you %s"%(username, greeting))
Các hàm có thể trả về một giá trị cho người gọi sử dụng từ khóa 'return'. Ví dụ:
def sum_two_numbers(a, b):
return a + b
Làm thế nào để gọi hàm trong Python?
Chỉ cần viết tên của hàm kèm theo (), đặt mọi đối số cần thiết trong dấu ngoặc. Ví dụ, hãy gọi các hàm đã viết ở trên (trong ví dụ trước):
# Define our 3 functions
def my_function():
print("Hello From My Function!")
def my_function_with_args(username, greeting):
print("Hello, %s, From My Function!, I wish you %s"%(username, greeting))
def sum_two_numbers(a, b):
return a + b
# print(a simple greeting)
my_function()
#prints - "Hello, John Doe, From My Function!, I wish you a great year!"
my_function_with_args("John Doe", "a great year!")
# after this line x will hold the value 3!
x = sum_two_numbers(1,2)
Exercise
Trong bài tập này, bạn sẽ sử dụng một hàm hiện có, và thêm hàm của riêng bạn để tạo một chương trình hoàn chỉnh.
-
Thêm một hàm tên là
list_benefits()
trả về danh sách sau: "More organized code", "More readable code", "Easier code reuse", "Allowing programmers to share and connect code together" -
Thêm một hàm tên là
build_sentence(info)
nhận một đối số chứa một chuỗi và trả về một câu bắt đầu với chuỗi đã cho và kết thúc bằng " is a benefit of functions!" -
Chạy và xem tất cả các hàm cùng hoạt động!
# Modify this function to return a list of strings as defined above
def list_benefits():
return []
# Modify this function to concatenate to each benefit - " is a benefit of functions!"
def build_sentence(benefit):
return ""
def name_the_benefits_of_functions():
list_of_benefits = list_benefits()
for benefit in list_of_benefits:
print(build_sentence(benefit))
name_the_benefits_of_functions()
# Modify this function to return a list of strings as defined above
def list_benefits():
return "More organized code", "More readable code", "Easier code reuse", "Allowing programmers to share and connect code together"
# Modify this function to concatenate to each benefit - " is a benefit of functions!"
def build_sentence(benefit):
return "%s is a benefit of functions!" % benefit
def name_the_benefits_of_functions():
list_of_benefits = list_benefits()
for benefit in list_of_benefits:
print(build_sentence(benefit))
name_the_benefits_of_functions()
test_output_contains("More organized code is a benefit of functions!")
test_output_contains("More readable code is a benefit of functions!")
test_output_contains("Easier code reuse is a benefit of functions!")
test_output_contains("Allowing programmers to share and connect code together is a benefit of functions!")
success_msg("Nice work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
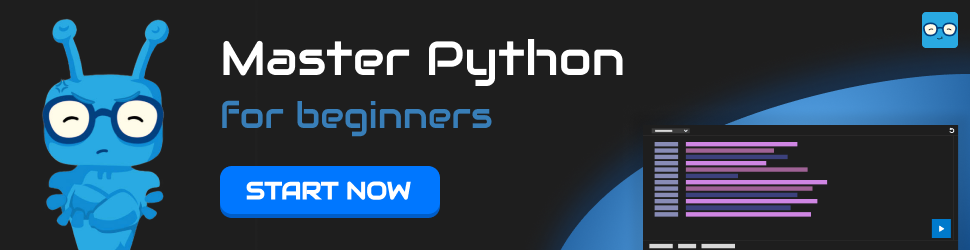