Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
الفئات والكائنات
الأشياء هي تجسيد للمتغيرات والوظائف في كيان واحد. تحصل الأشياء على متغيراتها ووظائفها من الفئات. الفئات هي في الأساس قالب لإنشاء أشيائك.
فئة بسيطة جدًا ستبدو شيئًا مثل هذا:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
سنشرح لاحقًا لماذا يجب عليك تضمين "self" كمعامل قليلاً. أولاً، لتعيين الفئة أعلاه (القالب) إلى كائن ستقوم بالآتي:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
الآن المتغير "myobjectx" يحمل كائنًا من الفئة "MyClass" يحتوي على المتغير والوظيفة المحددة داخل الفئة المسماة "MyClass".
الوصول إلى متغيرات الكائن
للوصول إلى المتغير داخل الكائن الجديد "myobjectx" ستقوم بالآتي:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
myobjectx.variable
لذلك على سبيل المثال، فإن الكود أدناه سيُخرج السلسلة النصية "blah":
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
print(myobjectx.variable)
يمكنك إنشاء عدة كائنات مختلفة تنتمي إلى نفس الفئة (تحتوي على نفس المتغيرات والوظائف المحددة). ومع ذلك، كل كائن يحتوي على نسخ مستقلة من المتغيرات المحددة في الفئة. على سبيل المثال، إذا كنا نريد تعريف كائن آخر باستخدام الفئة "MyClass" ثم نغير السلسلة النصية في المتغير أعلاه:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
myobjecty = MyClass()
myobjecty.variable = "yackity"
# ثم طبع كلا القيمتين
print(myobjectx.variable)
print(myobjecty.variable)
الوصول إلى وظائف الكائن
للوصول إلى وظيفة داخل كائن، تستخدم نفس النمط الذي يستخدم للوصول إلى متغير:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
myobjectx.function()
ما سبق سوف يطبع الرسالة: "This is a message inside the class."
init()
دالة __init__()
، هي دالة خاصة تُستدعى عند إنشاء الفئة.
تُستخدم لتعيين القيم في الفئة.
class NumberHolder:
def __init__(self, number):
self.number = number
def returnNumber(self):
return self.number
var = NumberHolder(7)
print(var.returnNumber()) # يطبع '7'
تمرين
لدينا فئة معرفة للمركبات. قم بإنشاء مركبتين جديدتين تسمى car1 و car2. حدد car1 ليكون سيار حمراء قابلة للتحويل تقدر بـ 60,000.00 دولار باسم Fer، و car2 لتكون شاحنة زرقاء باسم Jump تقدر بـ 10,000.00 دولار.
# define the Vehicle class
class Vehicle:
name = ""
kind = "car"
color = ""
value = 100.00
def description(self):
desc_str = "%s is a %s %s worth $%.2f." % (self.name, self.color, self.kind, self.value)
return desc_str
# your code goes here
# test code
print(car1.description())
print(car2.description())
# define the Vehicle class
class Vehicle:
name = ""
kind = "car"
color = ""
value = 100.00
def description(self):
desc_str = "%s is a %s %s worth $%.2f." % (self.name, self.color, self.kind, self.value)
return desc_str
# your code goes here
car1 = Vehicle()
car1.name = "Fer"
car1.color = "red"
car1.kind = "convertible"
car1.value = 60000.00
car2 = Vehicle()
car2.name = "Jump"
car2.color = "blue"
car2.kind = "van"
car2.value = 10000.00
# test code
print(car1.description())
print(car2.description())
#test_output_contains('Fer is a red convertible worth $60000.00.')
#test_output_contains('Jump is a blue van worth $10000.00.')
success_msg("Great job!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
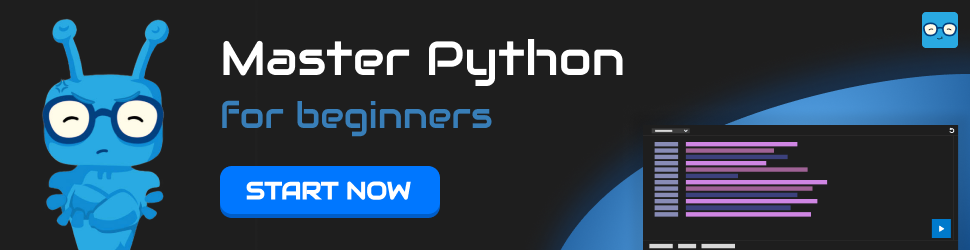