Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
التسلسل
Python توفر مكتبات JSON مدمجة لتشفير وفك تشفير JSON.
في Python 2.5، يتم استخدام وحدة simplejson، بينما في Python 2.7، يتم استخدام وحدة json. بما أن هذا المفسر يستخدم Python 2.7، فسنستخدم وحدة json.
من أجل استخدام وحدة json، يجب أولاً استيرادها:
import json
هناك صيغتان أساسيتان لبيانات JSON. إما أن تكون في شكل سلسلة نصية أو في شكل بنية بيانات موضوعية. في Python، تتكون بنية البيانات الموضوعية من القوائم والقواميس المتداخلة داخل بعضها البعض. بنية البيانات الموضوعية تتيح استخدام طرق بايثون (للقوائم والقواميس) لزيادة وتعداد والبحث وإزالة العناصر من بنية البيانات. صيغة السلسلة النصية تُستخدم بشكل رئيسي لتمرير البيانات إلى برنامج آخر أو لتحميلها إلى بنية بيانات.
لتحميل JSON مرة أخرى إلى بنية بيانات، استخدم طريقة "loads". هذه الطريقة تأخذ سلسلة نصية وتحولها مرة أخرى إلى بنية بيانات json:
import json
print(json.loads(json_string))
لتشفير بنية بيانات إلى JSON، استخدم طريقة "dumps". هذه الطريقة تأخذ كائنًا وتعيد سلسلة نصية:
import json
json_string = json.dumps([1, 2, 3, "a", "b", "c"])
print(json_string)
Python تدعم طريقة تسلسل بيانات ملكية بايثون تسمى pickle (ويوجد بديل أسرع يُدعى cPickle).
يمكنك استخدامها بنفس الطريقة تمامًا.
import pickle
pickled_string = pickle.dumps([1, 2, 3, "a", "b", "c"])
print(pickle.loads(pickled_string))
الهدف من هذا التمرين هو طباعة سلسلة JSON مع إضافة زوج المفتاح والقيمة "Me" : 800 إليها.
Exercise--------
import json
# fix this function, so it adds the given name
# and salary pair to salaries_json, and return it
def add_employee(salaries_json, name, salary):
# Add your code here
return salaries_json
# test code
salaries = '{"Alfred" : 300, "Jane" : 400 }'
new_salaries = add_employee(salaries, "Me", 800)
decoded_salaries = json.loads(new_salaries)
print(decoded_salaries["Alfred"])
print(decoded_salaries["Jane"])
print(decoded_salaries["Me"])
import json
# fix this function, so it adds the given name
# and salary pair to salaries_json, and return it
def add_employee(salaries_json, name, salary):
salaries = json.loads(salaries_json)
salaries[name] = salary
return json.dumps(salaries)
# test code
salaries = '{"Alfred" : 300, "Jane" : 400 }'
new_salaries = add_employee(salaries, "Me", 800)
decoded_salaries = json.loads(new_salaries)
print(decoded_salaries["Alfred"])
print(decoded_salaries["Jane"])
print(decoded_salaries["Me"])
test_output_contains("300")
test_output_contains("400")
test_output_contains("800")
success_msg("Great work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
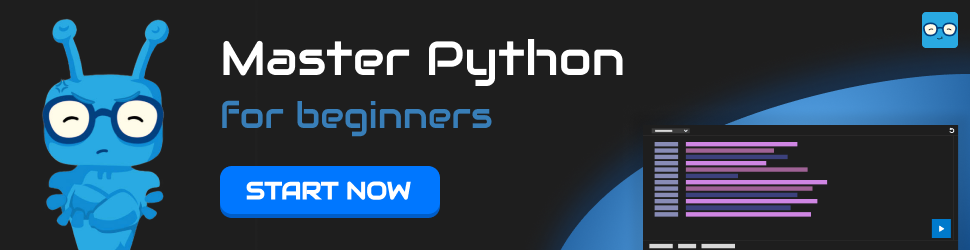