Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Silmukat
Pythonissa on kaksi tyyppiä silmukoita, for ja while.
The "for" loop
For-silmukat iteroivat annetun sekvenssin yli. Tässä on esimerkki:
primes = [2, 3, 5, 7]
for prime in primes:
print(prime)
For-silmukat voivat iteroida numerosekvenssin yli käyttämällä "range"- ja "xrange"-funktioita. Erona range- ja xrange-funktioiden välillä on se, että range palauttaa uuden listan, jossa on määrätyn alueen numerot, kun taas xrange palauttaa iteraattorin, joka on tehokkaampi. (Python 3 käyttää range-funktiota, joka toimii kuten xrange). Huomaa, että range-funktio on nollapohjainen.
# Tulostaa numerot 0,1,2,3,4
for x in range(5):
print(x)
# Tulostaa 3,4,5
for x in range(3, 6):
print(x)
# Tulostaa 3,5,7
for x in range(3, 8, 2):
print(x)
"while" loops
While-silmukat toistavat niin kauan kuin tietty ehto on voimassa. Esimerkiksi:
# Tulostaa 0,1,2,3,4
count = 0
while count < 5:
print(count)
count += 1 # Tämä on sama kuin count = count + 1
"break" ja "continue" lauseet
break:iä käytetään poistumaan for- tai while-silmukasta, kun taas continue:a käytetään ohittamaan nykyinen lohko ja palaamaan "for"- tai "while"-lausekkeeseen. Muutama esimerkki:
# Tulostaa 0,1,2,3,4
count = 0
while True:
print(count)
count += 1
if count >= 5:
break
# Tulostaa vain parittomat numerot - 1,3,5,7,9
for x in range(10):
# Tarkista, onko x parillinen
if x % 2 == 0:
continue
print(x)
Voimmeko käyttää "else" ehtolausetta silmukoille?
Toisin kuin kielissä kuten C, CPP.. voimme käyttää else silmukoille. Kun "for"- tai "while"-lausekkeen silmukkaehto epäonnistuu, silloin "else"-osassa oleva koodi suoritetaan. Jos break-lause suoritetaan silmukan sisällä, "else"-osa ohitetaan. Huomaa, että "else"-osa suoritetaan myös, vaikka käytössä on continue-lause.
Tässä on muutamia esimerkkejä:
# Tulostaa 0,1,2,3,4 ja sitten tulostaa "count value reached 5"
count=0
while(count<5):
print(count)
count +=1
else:
print("count value reached %d" %(count))
# Tulostaa 1,2,3,4
for i in range(1, 10):
if(i%5==0):
break
print(i)
else:
print("this is not printed because for loop is terminated because of break but not due to fail in condition")
Harjoitus
Käy läpi ja tulosta kaikki parilliset numerot numbers-listasta samassa järjestyksessä kuin ne vastaanotetaan. Älä tulosta mitään numeroita, jotka tulevat 237:n jälkeen sekvenssissä.
numbers = [
951, 402, 984, 651, 360, 69, 408, 319, 601, 485, 980, 507, 725, 547, 544,
615, 83, 165, 141, 501, 263, 617, 865, 575, 219, 390, 984, 592, 236, 105, 942, 941,
386, 462, 47, 418, 907, 344, 236, 375, 823, 566, 597, 978, 328, 615, 953, 345,
399, 162, 758, 219, 918, 237, 412, 566, 826, 248, 866, 950, 626, 949, 687, 217,
815, 67, 104, 58, 512, 24, 892, 894, 767, 553, 81, 379, 843, 831, 445, 742, 717,
958, 609, 842, 451, 688, 753, 854, 685, 93, 857, 440, 380, 126, 721, 328, 753, 470,
743, 527
]
# your code goes here
for number in numbers:
numbers = [
951, 402, 984, 651, 360, 69, 408, 319, 601, 485, 980, 507, 725, 547, 544,
615, 83, 165, 141, 501, 263, 617, 865, 575, 219, 390, 984, 592, 236, 105, 942, 941,
386, 462, 47, 418, 907, 344, 236, 375, 823, 566, 597, 978, 328, 615, 953, 345,
399, 162, 758, 219, 918, 237, 412, 566, 826, 248, 866, 950, 626, 949, 687, 217,
815, 67, 104, 58, 512, 24, 892, 894, 767, 553, 81, 379, 843, 831, 445, 742, 717,
958, 609, 842, 451, 688, 753, 854, 685, 93, 857, 440, 380, 126, 721, 328, 753, 470,
743, 527
]
# your code goes here
for number in numbers:
if number == 237:
break
if number % 2 == 1:
continue
print(number)
test_object("number", undefined_msg="Define a object `number` using the code from the tutorial to print just the desired numbers from the exercise description.",incorrect_msg="Your `number` object is not correct, You should use an `if` statement and a `break` statement to accomplish your goal.")
success_msg("Great work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
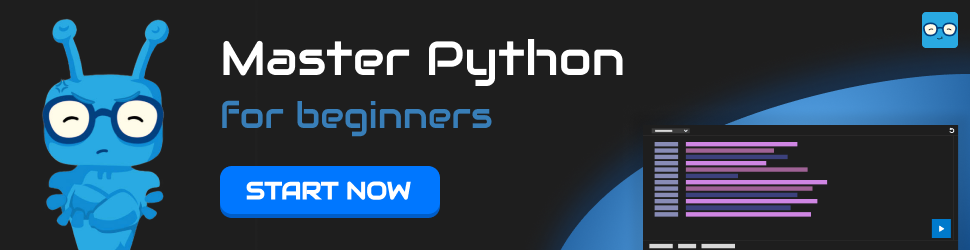