Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Funções
O que são Funções?
Funções são uma maneira conveniente de dividir seu código em blocos úteis, permitindo organizar o código, torná-lo mais legível, reutilizá-lo e economizar tempo. Além disso, funções são uma forma essencial de definir interfaces para que programadores possam compartilhar seu código.
Como escrever funções em Python?
Como vimos em tutoriais anteriores, Python utiliza blocos.
Um bloco é uma área de código escrita no formato de:
Onde uma linha de bloco é mais código Python (até mesmo outro bloco), e a cabeça do bloco tem o seguinte formato: block_keyword block_name(argument1,argument2, ...) Palavras-chave que você já conhece são "if", "for" e "while".
Funções em Python são definidas usando a palavra-chave de bloco "def", seguida pelo nome da função como o nome do bloco. Por exemplo:
Funções também podem receber argumentos (variáveis passadas do chamador para a função). Por exemplo:
Funções podem retornar um valor para o chamador, utilizando a palavra-chave 'return'. Por exemplo:
Como chamar funções em Python?
Basta escrever o nome da função seguido de (), colocando quaisquer argumentos necessários dentro dos parênteses. Por exemplo, vamos chamar as funções escritas acima (no exemplo anterior):
Exercício
Neste exercício, você usará uma função existente e adicionará a sua própria para criar um programa totalmente funcional.
-
Adicione uma função chamada
list_benefits()
que retorna a seguinte lista de strings: "Mais código organizado", "Código mais legível", "Reutilização de código mais fácil", "Permitindo que os programadores compartilhem e conectem código juntos" -
Adicione uma função chamada
build_sentence(info)
que recebe um único argumento contendo uma string e retorna uma sentença começando com a string fornecida e terminando com a string " é um benefício das funções!" -
Execute e veja todas as funções trabalhando juntas!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
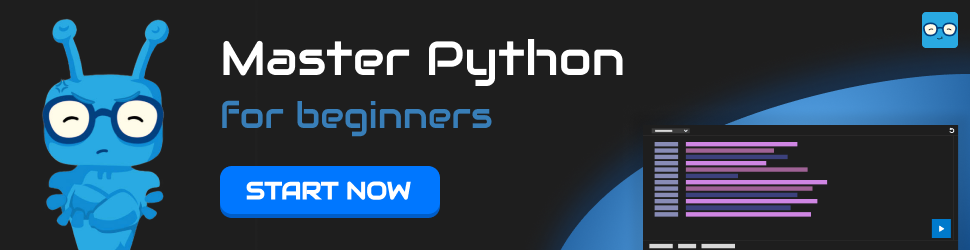