Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Laços
Existem dois tipos de loops em Python, for e while.
O loop "for"
Loops for iteram sobre uma sequência dada. Aqui está um exemplo:
Loops for podem iterar sobre uma sequência de números usando as funções "range" e "xrange". A diferença entre range e xrange é que a função range retorna uma nova lista com números daquele intervalo especificado, enquanto xrange retorna um iterador, que é mais eficiente. (Python 3 usa a função range, que age como xrange). Note que a função range é baseada em zero.
Loops "while"
Loops while repetem enquanto uma certa condição booleana é satisfeita. Por exemplo:
Instruções "break" e "continue"
break é usado para sair de um loop for ou de um loop while, enquanto continue é usado para pular o bloco atual e retornar à instrução "for" ou "while". Alguns exemplos:
Podemos usar cláusula "else" para loops?
Diferente de linguagens como C, CPP, podemos usar else em loops. Quando a condição do loop da instrução "for" ou "while" falha, então a parte do código em "else" é executada. Se uma instrução break for executada dentro do loop for, então a parte "else" é pulada. Note que a parte "else" é executada mesmo que haja uma instrução continue.
Aqui estão alguns exemplos:
Exercício
Percorra e imprima todos os números pares da lista de números na mesma ordem em que são recebidos. Não imprima nenhum número que venha após 237 na sequência.
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
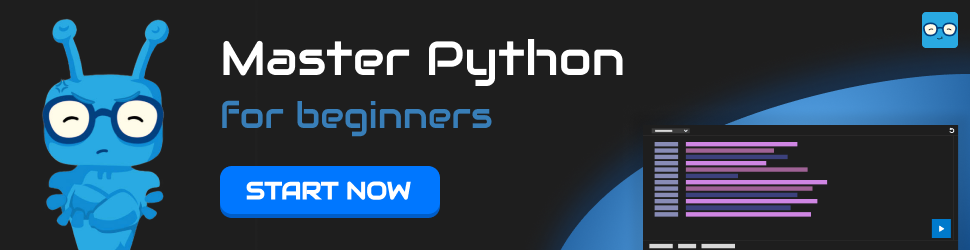