Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Temel Operatörler
Bu bölüm, Python'da temel operatörlerin nasıl kullanılacağını açıklar.
Aritmetik Operatörler
Diğer programlama dillerinde olduğu gibi, toplama, çıkarma, çarpma ve bölme operatörleri sayılarla kullanılabilir.
number = 1 + 2 * 3 / 4.0
print(number)
Sonucun ne olacağını tahmin etmeye çalışın. Python işlem önceliğine uyar mı?
Kullanılabilir diğer bir operatör ise, bölümün kalanını döndüren modül (%) operatörüdür. bölüm % bölen = kalan.
remainder = 11 % 3
print(remainder)
İki çarpma sembolü kullanmak bir üslü işlemi ifade eder.
squared = 7 ** 2
cubed = 2 ** 3
print(squared)
print(cubed)
Operatörleri Stringlerle Kullanma
Python, stringleri toplama operatörü kullanarak birleştirmeyi destekler:
helloworld = "hello" + " " + "world"
print(helloworld)
Python ayrıca, tekrarlayan bir dizilimle bir string oluşturmak için stringleri çarpmayı da destekler:
lotsofhellos = "hello" * 10
print(lotsofhellos)
Operatörleri Listelerle Kullanma
Listeler, toplama operatörleri ile birleştirilebilir:
even_numbers = [2,4,6,8]
odd_numbers = [1,3,5,7]
all_numbers = odd_numbers + even_numbers
print(all_numbers)
Stringlerde olduğu gibi, Python çarpma operatörünü kullanarak tekrarlayan bir dizilimle yeni listeler oluşturmayı da destekler:
print([1,2,3] * 3)
Egzersiz
Bu egzersizin hedefi, x_list
ve y_list
adında iki liste oluşturmaktır ve bu listeler, sırasıyla x
ve y
değişkenlerinin 10 örneğini içermelidir.
Ayrıca, oluşturduğunuz iki listeyi birleştirerek içinde x
ve y
değişkenlerinin her birinin 10 kez bulunduğu big_list
adında bir liste oluşturmanız gerekmektedir.
x = object()
y = object()
# TODO: change this code
x_list = [x]
y_list = [y]
big_list = []
print("x_list contains %d objects" % len(x_list))
print("y_list contains %d objects" % len(y_list))
print("big_list contains %d objects" % len(big_list))
# testing code
if x_list.count(x) == 10 and y_list.count(y) == 10:
print("Almost there...")
if big_list.count(x) == 10 and big_list.count(y) == 10:
print("Great!")
x = object()
y = object()
# TODO: change this code
x_list = [x] * 10
y_list = [y] * 10
big_list = x_list + y_list
print("x_list contains %d objects" % len(x_list))
print("y_list contains %d objects" % len(y_list))
print("big_list contains %d objects" % len(big_list))
# testing code
if x_list.count(x) == 10 and y_list.count(y) == 10:
print("Almost there...")
if big_list.count(x) == 10 and big_list.count(y) == 10:
print("Great!")
Ex().check_object('x_list').has_equal_value(expr_code = 'len(x_list)')
Ex().check_object('y_list').has_equal_value(expr_code = 'len(y_list)')
Ex().check_object('big_list').has_equal_value(expr_code = 'len(big_list)')
success_msg('Good work!')
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
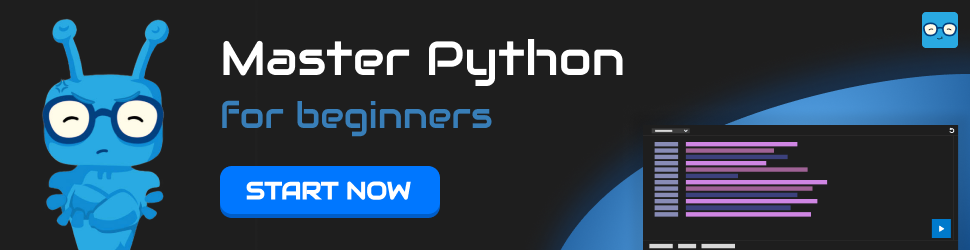