Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Düzenli İfadeler
Regular Expressions (sometimes shortened to regexp, regex, or re) are a
Regular ifadeler (bazen regexp, regex veya re olarak kısaltılır), metin içindeki desenleri eşleştirmek için bir araçtır. Python'da, re modülümüz vardır.
Düzenli ifadelerin uygulamaları geniş çapta yaygındır, ancak oldukça karmaşıktırlar, bu yüzden belli bir görev için bir regex kullanmayı düşünürken alternatifleri düşünün ve regexlere son çare olarak başvurun.
An example regex is `r"^(From|To|Cc).*[email protected]"` Now for an
Örnek bir regex `r"^(From|To|Cc).*[email protected]"` şeklindedir. Şimdi bir açıklama:
Kare işareti `^` metnin bir satırın başında eşleştiği anlamına gelir.
Takip eden grup, `(From|To|Cc)` ile olan kısım, satırın boru `|` ile ayrılan kelimelerden biriyle başlaması gerektiği anlamına gelir.
Bu, VEYA operatörü olarak adlandırılır ve grup içindeki kelimelerden herhangi biriyle başlarsa regex eşleşecektir.
`.*?`, satır sonu karakteri `\n` hariç herhangi bir sayıda karakterle eşleşmek anlamına gelir.
Aç gözlü olmayan kısım, mümkün olan en az tekrarı eşleştirmek anlamına gelir.
`.` karakteri, satır sonu olmayan herhangi bir karakter anlamına gelir, `*` 0 veya daha fazla kez tekrarlamak anlamına gelir ve `?` karakteri aç gözlü olmamasını sağlar.
So, the following lines would be matched by that regex:
Dolayısıyla, aşağıdaki satırlar bu regex tarafından eşleştirilecektir:
`From: [email protected]`
`To: !asp]<,. [email protected]`
A complete reference for the re syntax is available at the [python
Düzenli ifade sözdizimi için tam bir referans [python dokümanlarında](http://docs.python.org/library/re.html#regular-expression-syntax "RE syntax") bulunmaktadır.
As an example of a "proper" email-matching regex (like the one in the
Doğru bir e-posta eşleştirme regexinin örneği olarak (alıştırmadaki gibi), [buraya](http://www.ex-parrot.com/pdw/Mail-RFC822-Address.html) bakın.
# Example:
import re
pattern = re.compile(r"\[(on|off)\]") # Slight optimization
print(re.search(pattern, "Mono: Playback 65 [75%] [-16.50dB] [on]"))
# Returns a Match object!
print(re.search(pattern, "Nada...:-("))
# Doesn't return anything.
# End Example
# Exercise: make a regular expression that will match an email
def test_email(your_pattern):
pattern = re.compile(your_pattern)
emails = ["[email protected]", "[email protected]", "wha.t.`1an?ug{}[email protected]"]
for email in emails:
if not re.match(pattern, email):
print("You failed to match %s" % (email))
elif not your_pattern:
print("Forgot to enter a pattern!")
else:
print("Pass")
pattern = r"" # Your pattern here!
test_email(pattern)
# Exercise: make a regular expression that will match an email
import re
def test_email(your_pattern):
pattern = re.compile(your_pattern)
emails = ["[email protected]", "[email protected]", "wha.t.`1an?ug{}[email protected]"]
for email in emails:
if not re.match(pattern, email):
print("You failed to match %s" % (email))
elif not your_pattern:
print("Forgot to enter a pattern!")
else:
print("Pass")
# Your pattern here!
pattern = r"\"?([-a-zA-Z0-9.`?{}]+@\w+\.\w+)\"?"
test_email(pattern)
test_output_contains("Pass")
success_msg("Great work!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
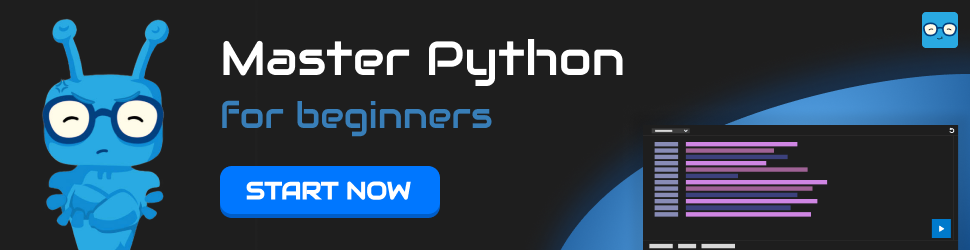