Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Класи та об'єкти
Об'єкти є інкапсуляцією змінних та функцій в одній сутності. Об'єкти беруть свої змінні та функції з класів. Класи, по суті, є шаблоном для створення ваших об'єктів.
Дуже базовий клас виглядає приблизно так:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
Ми пояснимо, чому вам потрібно включати цей "self" як параметр трохи пізніше. Спочатку, щоб призначити наведений вище клас (шаблон) для об'єкта, ви б зробили наступне:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
Тепер змінна "myobjectx" містить об'єкт класу "MyClass", який містить змінну та функцію, визначені в класі, що називається "MyClass".
Доступ до змінних об'єкта
Щоб отримати доступ до змінної всередині новоствореного об'єкта "myobjectx", ви зробите наступне:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
myobjectx.variable
Наприклад, нижче виведе рядок "blah":
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
print(myobjectx.variable)
Ви можете створити кілька різних об'єктів, які є того ж класу (мають такі ж змінні та функції, що визначені). Однак кожен об'єкт містить незалежні копії змінних, визначених у класі. Наприклад, якщо ми були б визначити інший об'єкт з класом "MyClass", а потім змінити рядок у змінній:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
myobjecty = MyClass()
myobjecty.variable = "yackity"
# Тоді виведемо обидва значення
print(myobjectx.variable)
print(myobjecty.variable)
Доступ до функцій об'єкта
Щоб отримати доступ до функції всередині об'єкта, ви використовуєте подібну нотацію до доступу до змінної:
class MyClass:
variable = "blah"
def function(self):
print("This is a message inside the class.")
myobjectx = MyClass()
myobjectx.function()
Наведене вище виведе повідомлення: "This is a message inside the class."
init()
Функція __init__()
, є спеціальною функцією, яка викликається, коли клас ініціюється.
Вона використовується для присвоєння значень у класі.
class NumberHolder:
def __init__(self, number):
self.number = number
def returnNumber(self):
return self.number
var = NumberHolder(7)
print(var.returnNumber()) # Виведе '7'
Exercise
Ми маємо клас, визначений для транспортних засобів. Створіть два нових транспортних засоби під назвою car1 та car2. Встановіть car1 як червоний кабріолет вартістю $60,000.00 з ім'ям Fer, а car2 як синій фургон на ім'я Jump вартістю $10,000.00.
# define the Vehicle class
class Vehicle:
name = ""
kind = "car"
color = ""
value = 100.00
def description(self):
desc_str = "%s is a %s %s worth $%.2f." % (self.name, self.color, self.kind, self.value)
return desc_str
# your code goes here
# test code
print(car1.description())
print(car2.description())
# define the Vehicle class
class Vehicle:
name = ""
kind = "car"
color = ""
value = 100.00
def description(self):
desc_str = "%s is a %s %s worth $%.2f." % (self.name, self.color, self.kind, self.value)
return desc_str
# your code goes here
car1 = Vehicle()
car1.name = "Fer"
car1.color = "red"
car1.kind = "convertible"
car1.value = 60000.00
car2 = Vehicle()
car2.name = "Jump"
car2.color = "blue"
car2.kind = "van"
car2.value = 10000.00
# test code
print(car1.description())
print(car2.description())
#test_output_contains('Fer is a red convertible worth $60000.00.')
#test_output_contains('Jump is a blue van worth $10000.00.')
success_msg("Great job!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
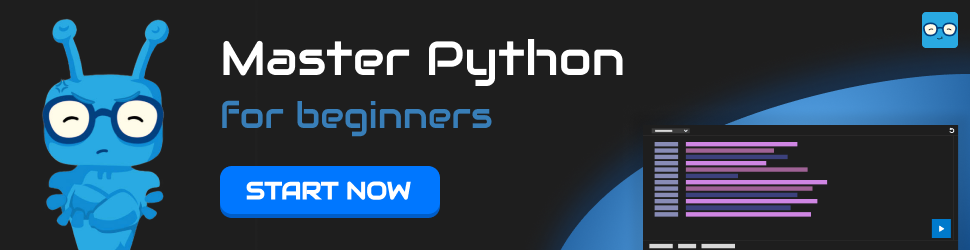