Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Змінні та типи
Python є повністю об'єктно-орієнтованою мовою програмування і не має "статичної типізації". Вам не потрібно оголошувати змінні перед їх використанням або оголошувати їх тип. Кожна змінна в Python є об'єктом.
Цей підручник розгляне кілька базових типів змінних.
Числа
Python підтримує два типи чисел - цілі числа (цілі числа) та числа з плаваючою комою (десяткові дроби). (Він також підтримує комплексні числа, які не будуть пояснюватися в цьому підручнику).
Щоб визначити ціле число, використовуйте наступний синтаксис:
myint = 7
print(myint)
Щоб визначити число з плаваючою точкою, ви можете використовувати один з наступних записів:
myfloat = 7.0
print(myfloat)
myfloat = float(7)
print(myfloat)
Рядки
Рядки визначаються або за допомогою одинарних, або подвійних лапок.
mystring = 'hello'
print(mystring)
mystring = "hello"
print(mystring)
Різниця між ними полягає в тому, що використання подвійних лапок полегшує включення апострофів (оскільки вони закрили б рядок, якщо використовувати одинарні лапки)
mystring = "Don't worry about apostrophes"
print(mystring)
Існують додаткові варіації визначення рядків, які полегшують включення таких речей, як символи повернення каретки, зворотні косі лінії та символи Unicode. Це виходить за рамки цього підручника, але вони описані в документації Python.
На числах і рядках можна виконувати прості оператори:
one = 1
two = 2
three = one + two
print(three)
hello = "hello"
world = "world"
helloworld = hello + " " + world
print(helloworld)
Присвоєння можна зробити більше ніж для однієї змінної "одночасно" на тій самій лінії так
a, b = 3, 4
print(a, b)
Змішування операторів між числами та рядками не підтримується:
# Це не спрацює!
one = 1
two = 2
hello = "hello"
print(one + two + hello)
Вправа
Мета цієї вправи – створити рядок, ціле число та число з плаваючою точкою. Рядок повинен називатися mystring
і містити слово "hello". Число з плаваючою точкою повинно називатися myfloat
і містити число 10.0, а ціле число повинно називатися myint
і містити число 20.
# change this code
mystring = None
myfloat = None
myint = None
# testing code
if mystring == "hello":
print("String: %s" % mystring)
if isinstance(myfloat, float) and myfloat == 10.0:
print("Float: %f" % myfloat)
if isinstance(myint, int) and myint == 20:
print("Integer: %d" % myint)
# change this code
mystring = "hello"
myfloat = 10.0
myint = 20
# testing code
if mystring == "hello":
print("String: %s" % mystring)
if isinstance(myfloat, float) and myfloat == 10.0:
print("Float: %f" % myfloat)
if isinstance(myint, int) and myint == 20:
print("Integer: %d" % myint)
test_object('mystring', incorrect_msg="Don't forget to change `mystring` to the correct value from the exercise description.")
test_object('myfloat', incorrect_msg="Don't forget to change `myfloat` to the correct value from the exercise description.")
test_object('myint', incorrect_msg="Don't forget to change `myint` to the correct value from the exercise description.")
test_output_contains("String: hello",no_output_msg= "Make sure your string matches exactly to the exercise desciption.")
test_output_contains("Float: 10.000000",no_output_msg= "Make sure your float matches exactly to the exercise desciption.")
test_output_contains("Integer: 20",no_output_msg= "Make sure your integer matches exactly to the exercise desciption.")
success_msg("Great job!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
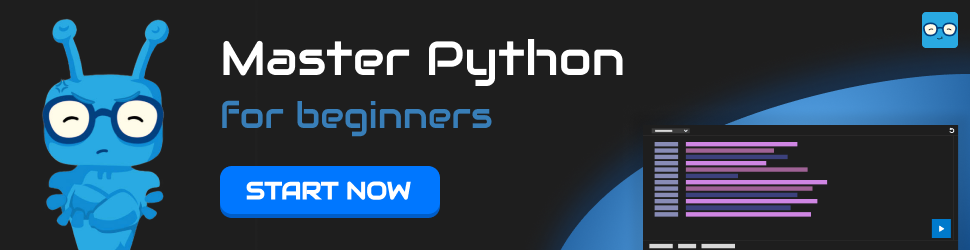