Get started learning Python with DataCamp's free Intro to Python tutorial. Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now!
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join 11 million other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount
Переменные и типы
Python является полностью объектно-ориентированным языком и не имеет "статической типизации". Вам не нужно объявлять переменные перед их использованием или указывать их тип. Каждая переменная в Python является объектом.
Этот учебник охватит несколько базовых типов переменных.
Numbers
Python поддерживает два типа чисел - целые числа и числа с плавающей точкой (десятичные дроби). (Он также поддерживает комплексные числа, которые в этом руководстве не рассматриваются).
Чтобы определить целое число, используйте следующий синтаксис:
myint = 7
print(myint)
Для определения числа с плавающей точкой вы можете использовать одну из следующих нотаций:
myfloat = 7.0
print(myfloat)
myfloat = float(7)
print(myfloat)
Strings
Строки определяются либо с помощью одиночных, либо двойных кавычек.
mystring = 'hello'
print(mystring)
mystring = "hello"
print(mystring)
Разница между ними в том, что использование двойных кавычек упрощает включение апострофов (поскольку они завершат строку при использовании одиночных кавычек).
mystring = "Don't worry about apostrophes"
print(mystring)
Существуют дополнительные вариации определения строк, которые упрощают включение таких вещей, как переносы строк, обратные слеши и символы Unicode. Они выходят за рамки этого руководства, но рассматриваются в документации Python.
На числах и строках можно выполнять простые операции:
one = 1
two = 2
three = one + two
print(three)
hello = "hello"
world = "world"
helloworld = hello + " " + world
print(helloworld)
Присваивания можно делать на более чем одной переменной "одновременно" на одной строке, как показано ниже:
a, b = 3, 4
print(a, b)
Перемешивание операторов между числами и строками не поддерживается:
# Это не сработает!
one = 1
two = 2
hello = "hello"
print(one + two + hello)
Exercise
Цель этого упражнения - создать строку, целое число и число с плавающей точкой. Строка должна называться mystring
и содержать слово "hello". Число с плавающей точкой должно называться myfloat
и содержать число 10.0, а целое число должно называться myint
и содержать число 20.
# change this code
mystring = None
myfloat = None
myint = None
# testing code
if mystring == "hello":
print("String: %s" % mystring)
if isinstance(myfloat, float) and myfloat == 10.0:
print("Float: %f" % myfloat)
if isinstance(myint, int) and myint == 20:
print("Integer: %d" % myint)
# change this code
mystring = "hello"
myfloat = 10.0
myint = 20
# testing code
if mystring == "hello":
print("String: %s" % mystring)
if isinstance(myfloat, float) and myfloat == 10.0:
print("Float: %f" % myfloat)
if isinstance(myint, int) and myint == 20:
print("Integer: %d" % myint)
test_object('mystring', incorrect_msg="Don't forget to change `mystring` to the correct value from the exercise description.")
test_object('myfloat', incorrect_msg="Don't forget to change `myfloat` to the correct value from the exercise description.")
test_object('myint', incorrect_msg="Don't forget to change `myint` to the correct value from the exercise description.")
test_output_contains("String: hello",no_output_msg= "Make sure your string matches exactly to the exercise desciption.")
test_output_contains("Float: 10.000000",no_output_msg= "Make sure your float matches exactly to the exercise desciption.")
test_output_contains("Integer: 20",no_output_msg= "Make sure your integer matches exactly to the exercise desciption.")
success_msg("Great job!")
This site is generously supported by DataCamp. DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
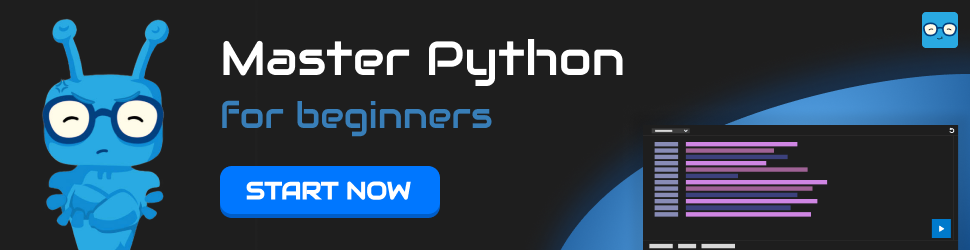